버튼
클릭하여 워크플로우를 실행하거나 페이지를 이동할 수 있습니다.
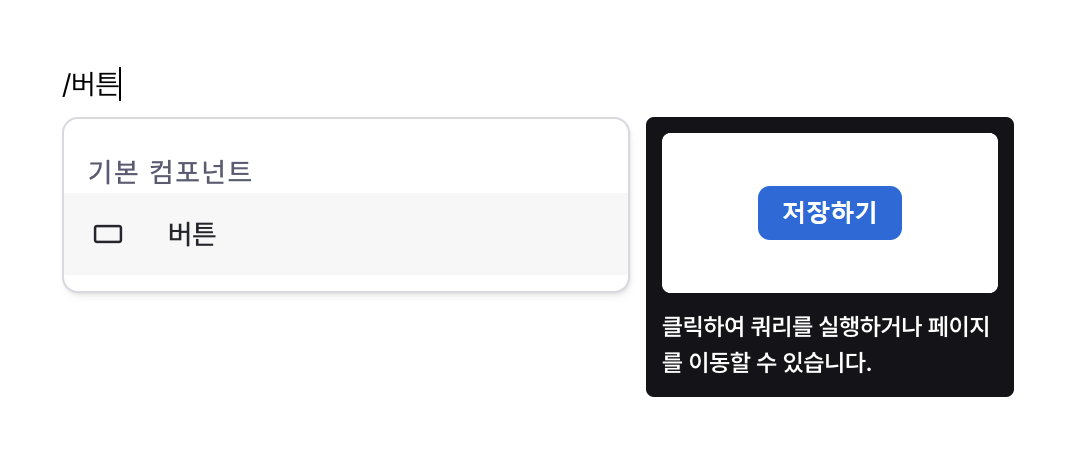
프로퍼티
프로퍼티 | 타입 | 설명 |
---|---|---|
이름 (name) | string | 버튼 컴포넌트의 고유 이름 |
레이블 (label) | string | 버튼에 표기되는 텍스트 |
실행 대상 (handler) | ActionHandler | 버튼 클릭 시 동작 정의 |
확인 모달 팝업 (confirmModal) | boolean | 버튼 클릭 시 확인 모달 여부 |
컴포넌트 비활성화 (isDisabled) | boolean | 버튼 비활성화 여부 |
컴포넌트 숨김 (isHidden) | boolean | 배포된 페이지에서 버튼 숨김 여부 |
버튼 색상 (color) | ButtonColor | 버튼의 색상 |
버튼 스타일 (variant) | ButtonVariant | 버튼의 스타일 |
이름 (name)
버튼 컴포넌트의 고유 이름을 입력할 수 있는 프로퍼티입니다. 이름 규칙을 참고해주세요.
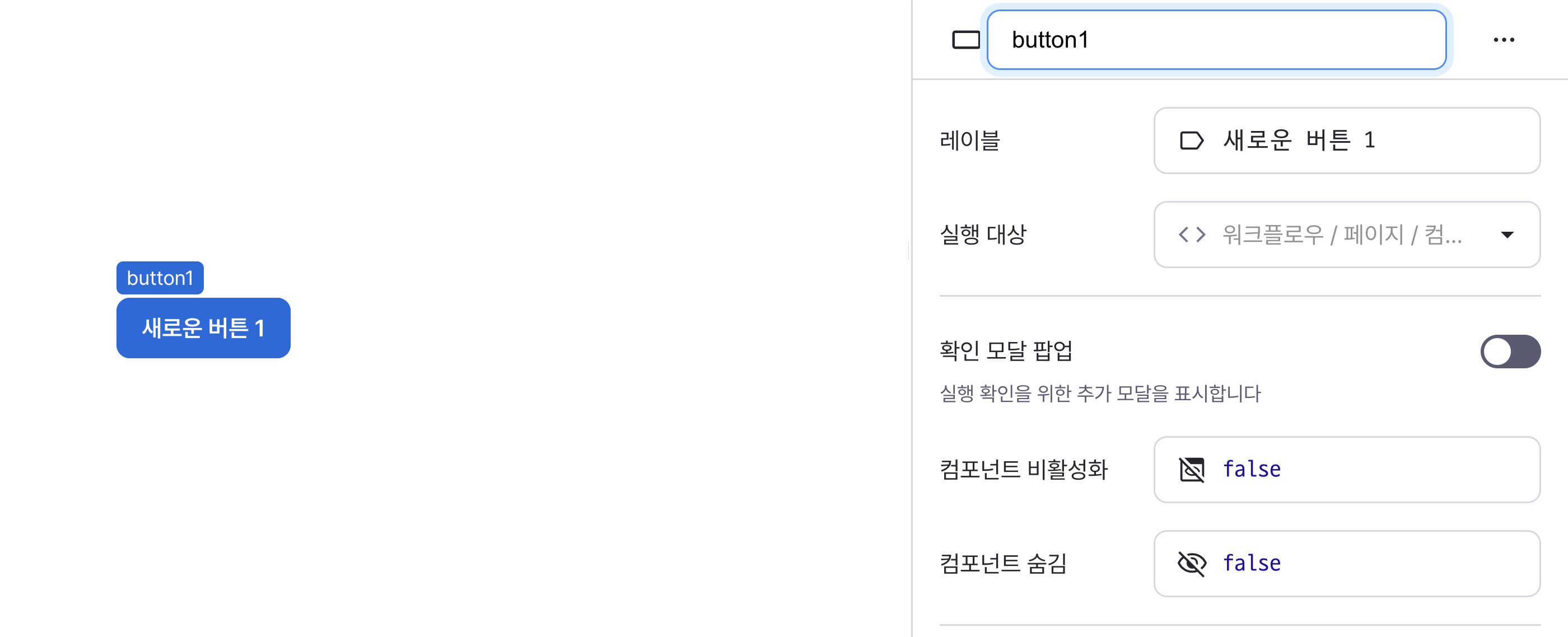
레이블 (label)
버튼에 표기되는 텍스트를 입력할 수 있는 프로퍼티입니다. (템플릿 텍스트 입력 가능)
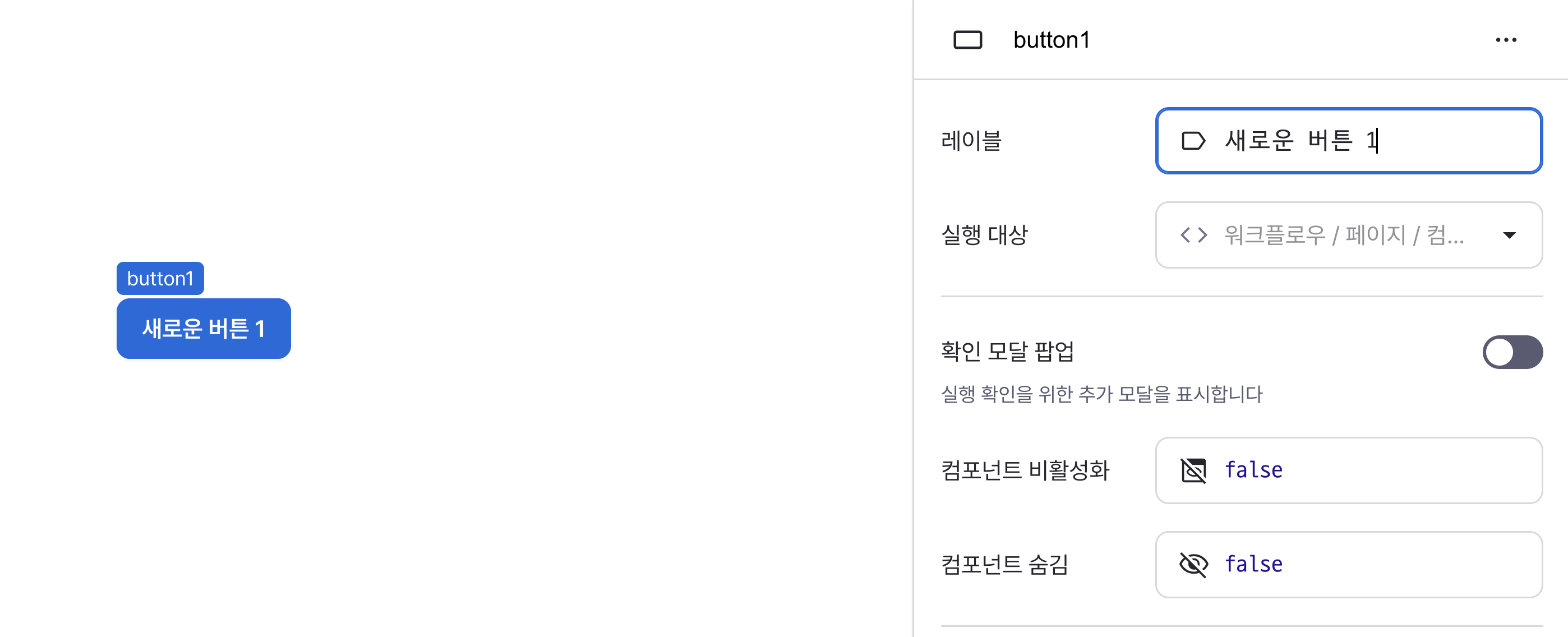
실행 대상 (handler)
버튼의 실행 대상을 선택할 수 있는 프로퍼티입니다. 버튼을 클릭했을 때 다음과 같은 실행 대상을 지정할 수 있습니다.
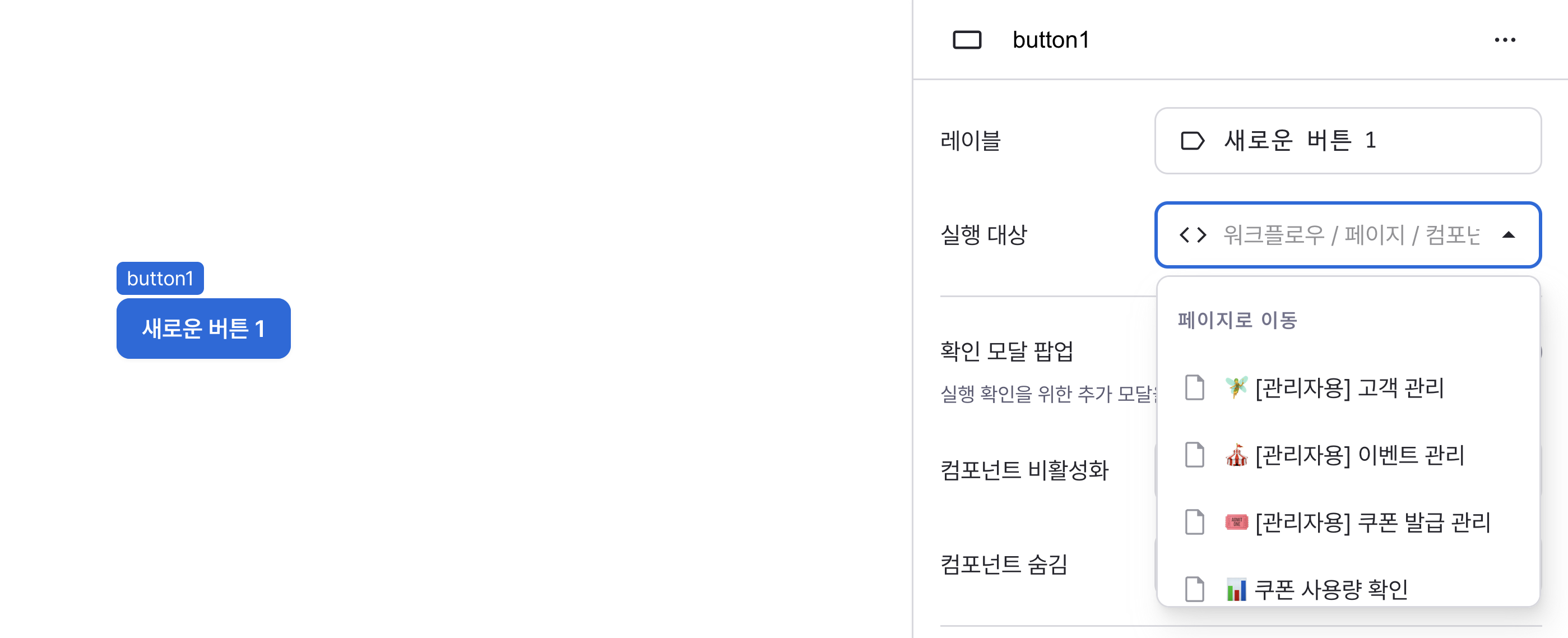
- 페이지로 이동
- 이동할 페이지를 선택할 수 있습니다.
- 선택한 페이지에 변수가 있다면 값을 전달할 수 있습니다.
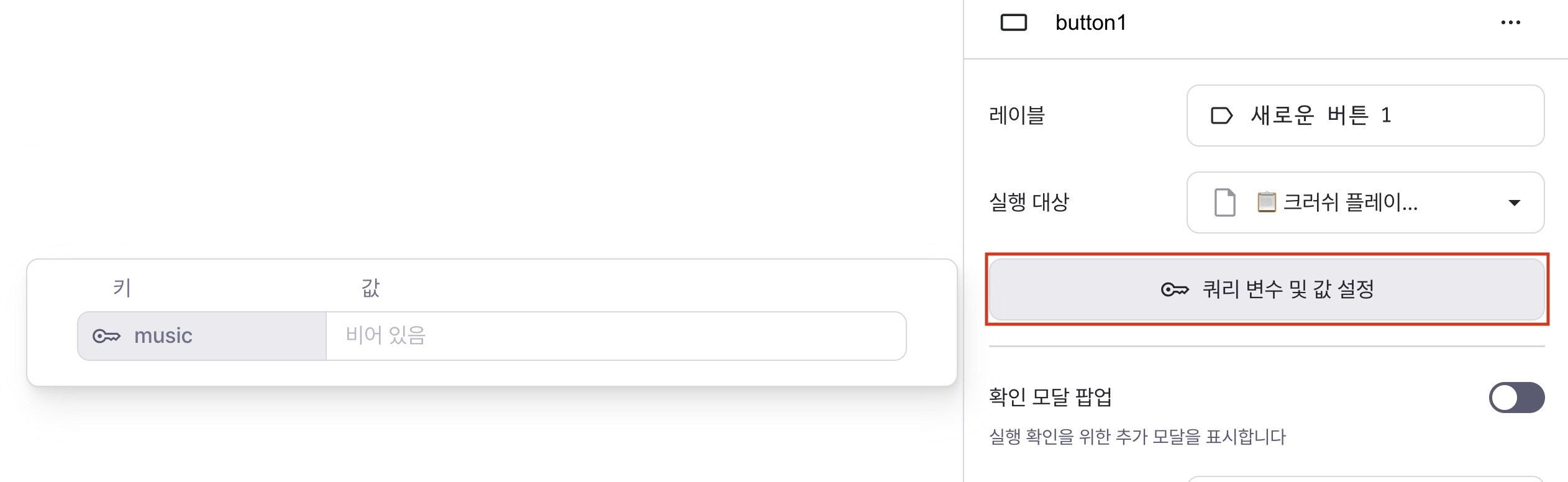
- 워크플로우 실행
- 실행할 워크플로우를 선택할 수 있습니다.
- 선택한 워크플로우에 변수가 있다면 값을 전달할 수 있습니다.
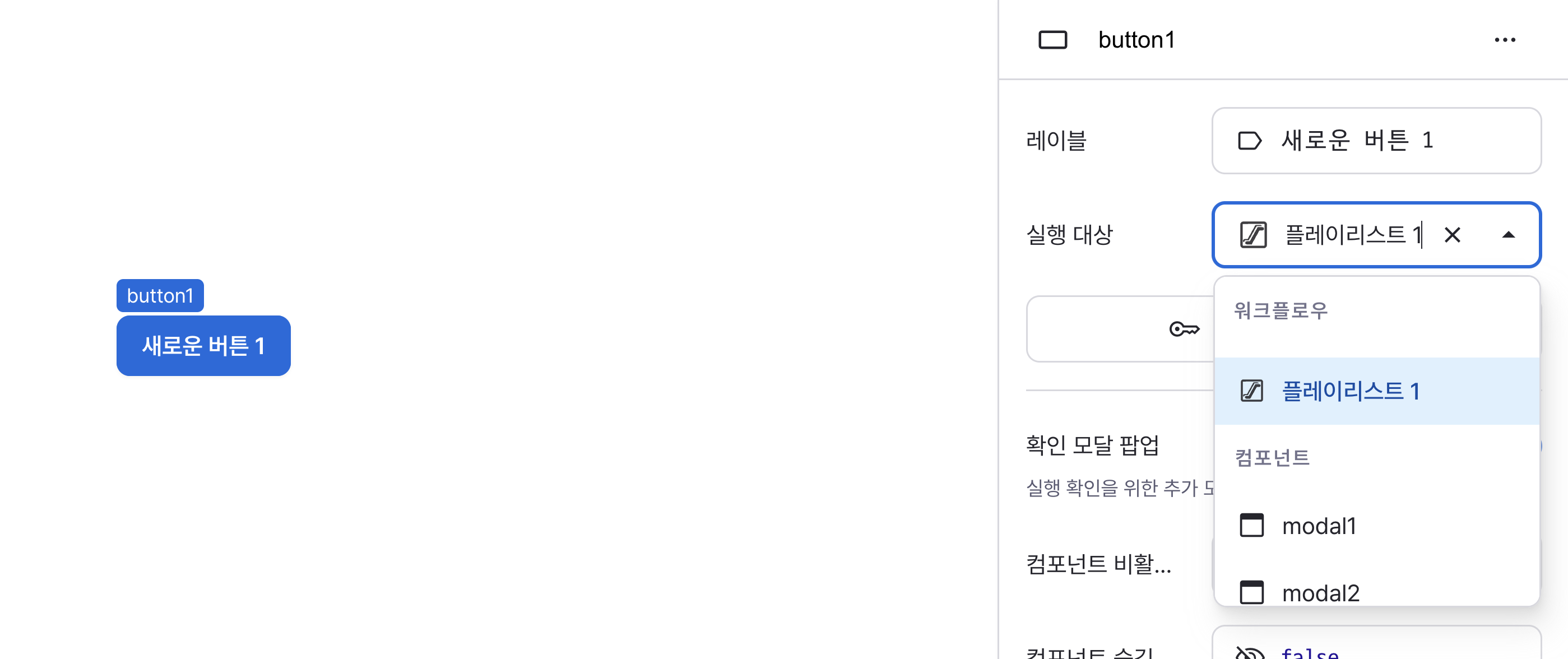
- (컴포넌트 동작 제어, 현재 모달 지원) 모달 열기 혹은 닫기
- 열거나 닫을 모달을 선택할 수 있습니다.
- 모달 여닫기 여부를 선택할 수 있습니다.
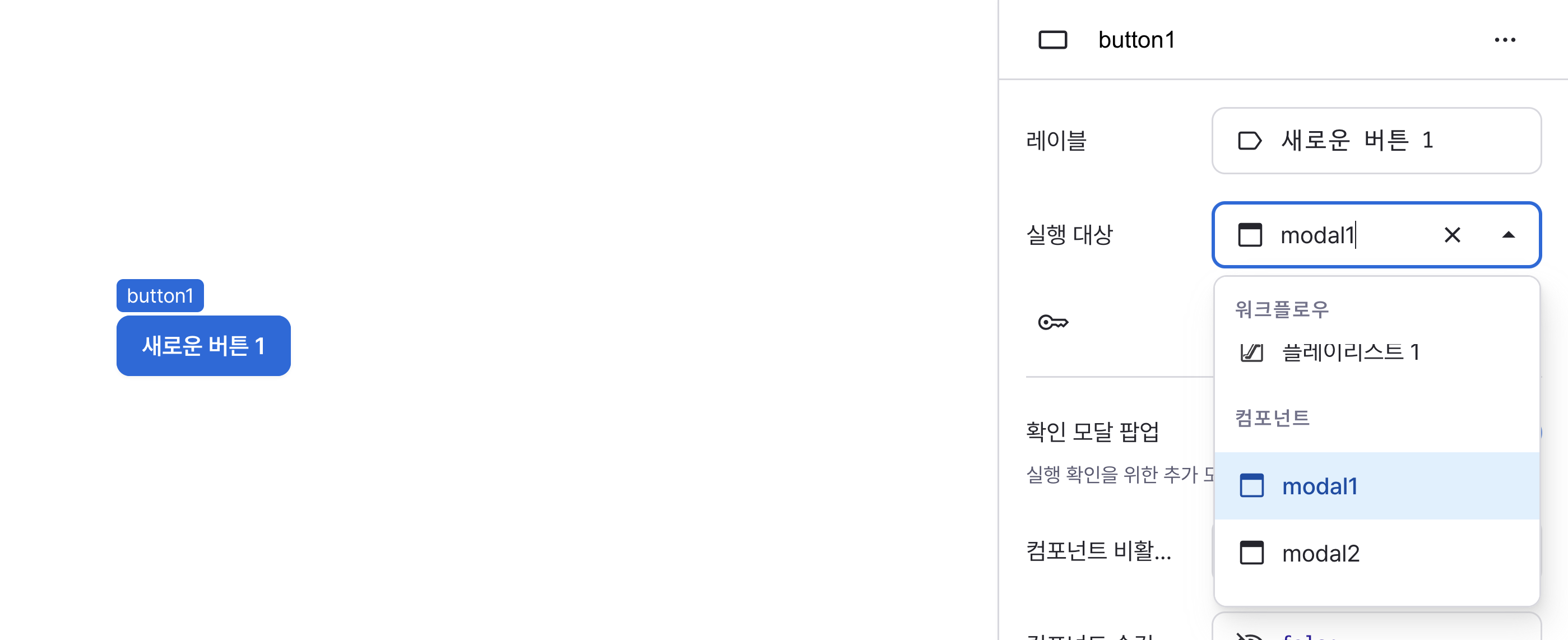
확인 모달 팝업 (confirmModal)
버튼 클릭 시 실행 대상 실행 전 확인 모달을 띄울지 정할 수 있는 프로퍼티입니다.
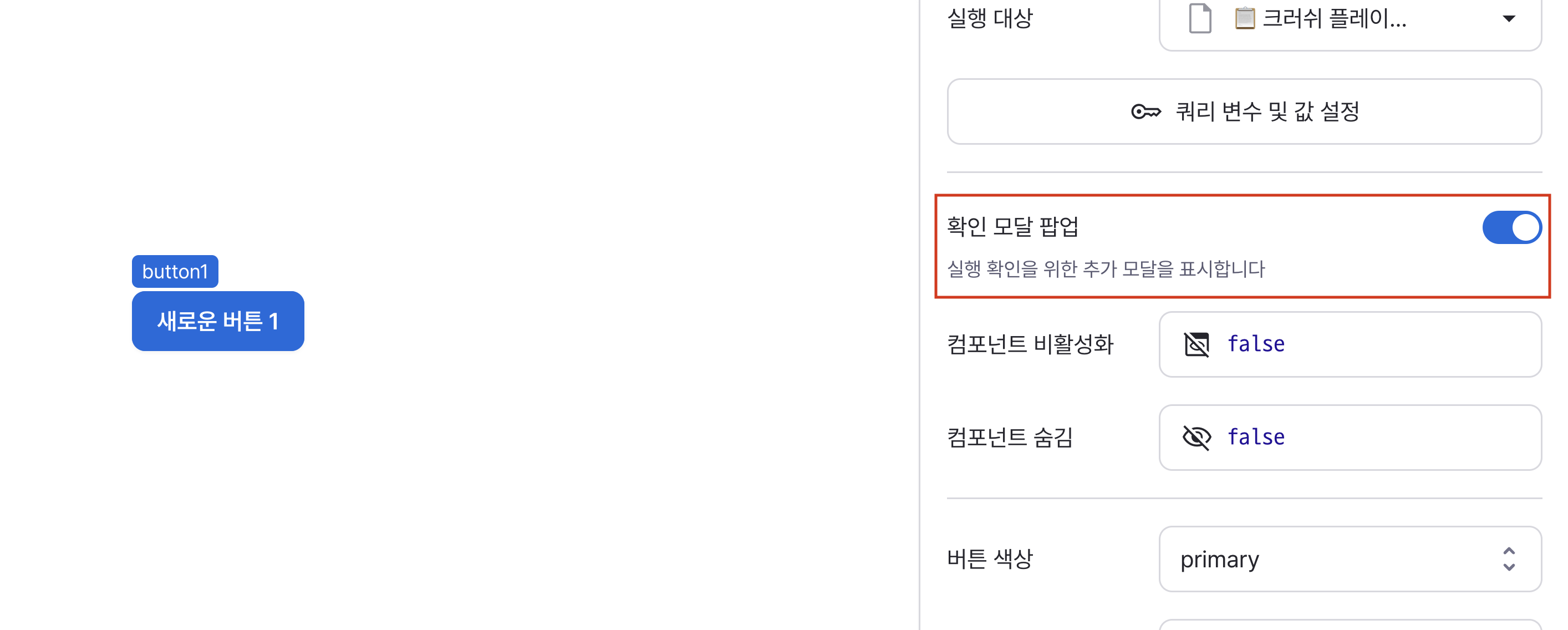
옵션 활성 시 버튼을 클릭 하면 아래와 같이 팝업 모달이 뜹니다.
삭제 기능과 같은 버튼 클릭을 신중하게 해야하는 경우 옵션을 추가해보세요.
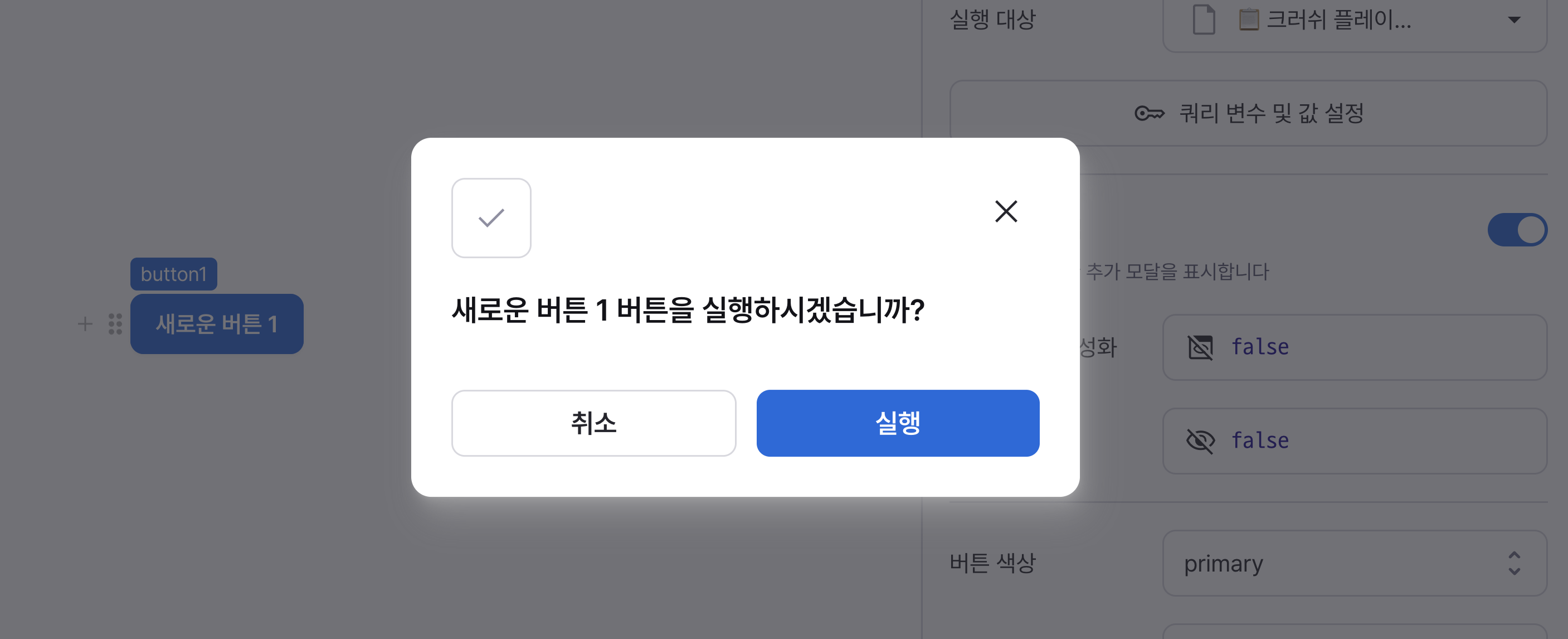
컴포넌트 비활성화 (isDisabled)
컴포넌트의 비활성화 상태를 입력하는 프로퍼티입니다.
워크플로우의 실행 결과를 사용하거나 직접 값을 입력하여 컴포넌트 비활성화 상태를 설정할 수 있습니다.
값을 true
로 설정할 경우, 해당 컴포넌트가 비활성화 상태로 변경됩니다.
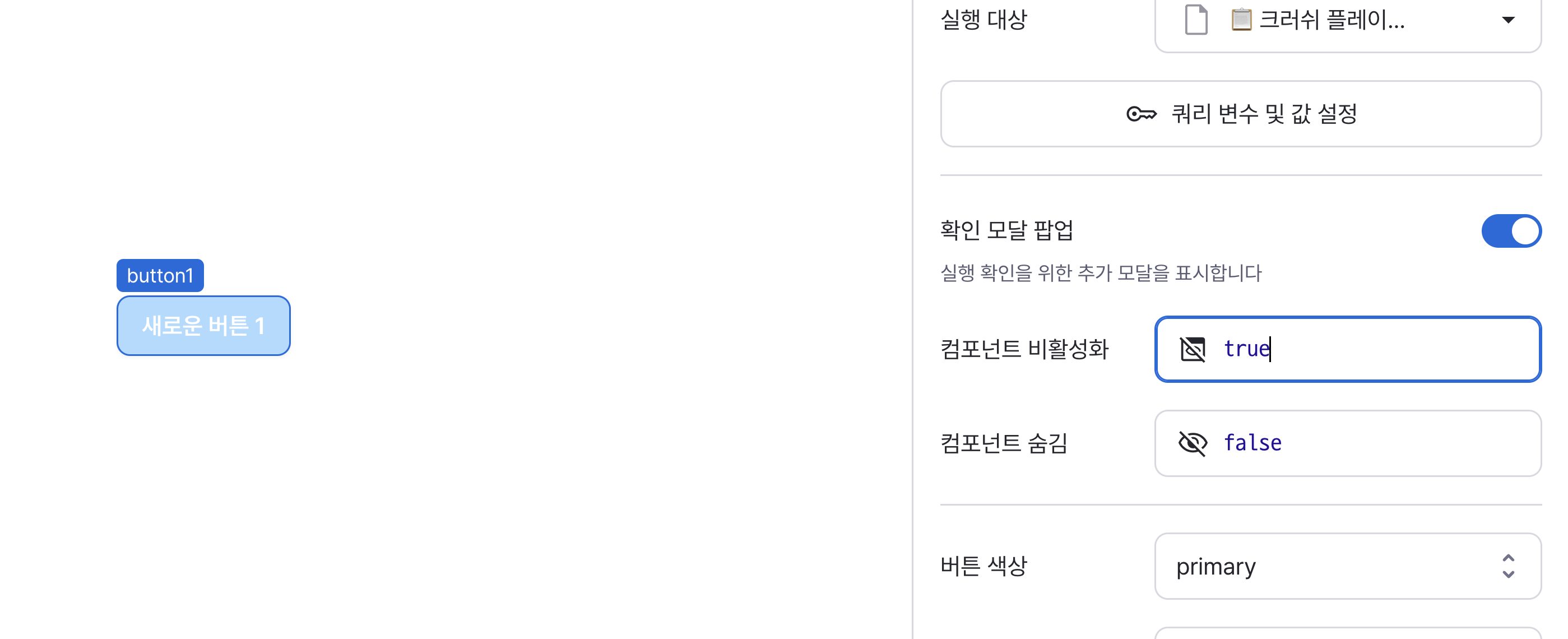
컴포넌트 숨김 (isHidden)
컴포넌트의 숨김 상태를 입력하는 프로퍼티입니다.
워크플로우의 실행 결과를 사용하거나 직접 값을 입력하여 컴포넌트 숨김 상태를 설정할 수 있습니다.
값을 true
로 설정할 경우, 배포된 상태에서는 컴포넌트가 숨겨지고 편집 상태에서는 불투명한 상태로 편집이 가능합니다.
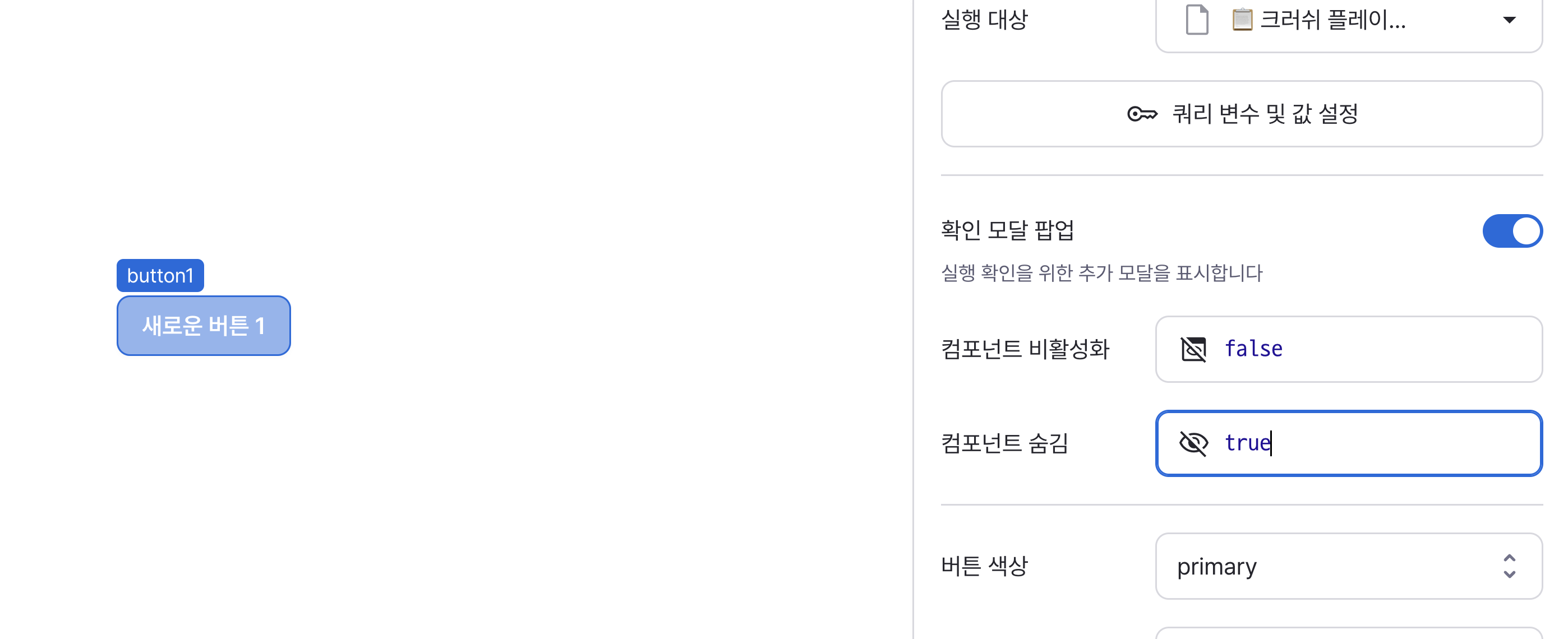
버튼 색상 (color)
버튼의 색상을 바꿀 수 있습니다.
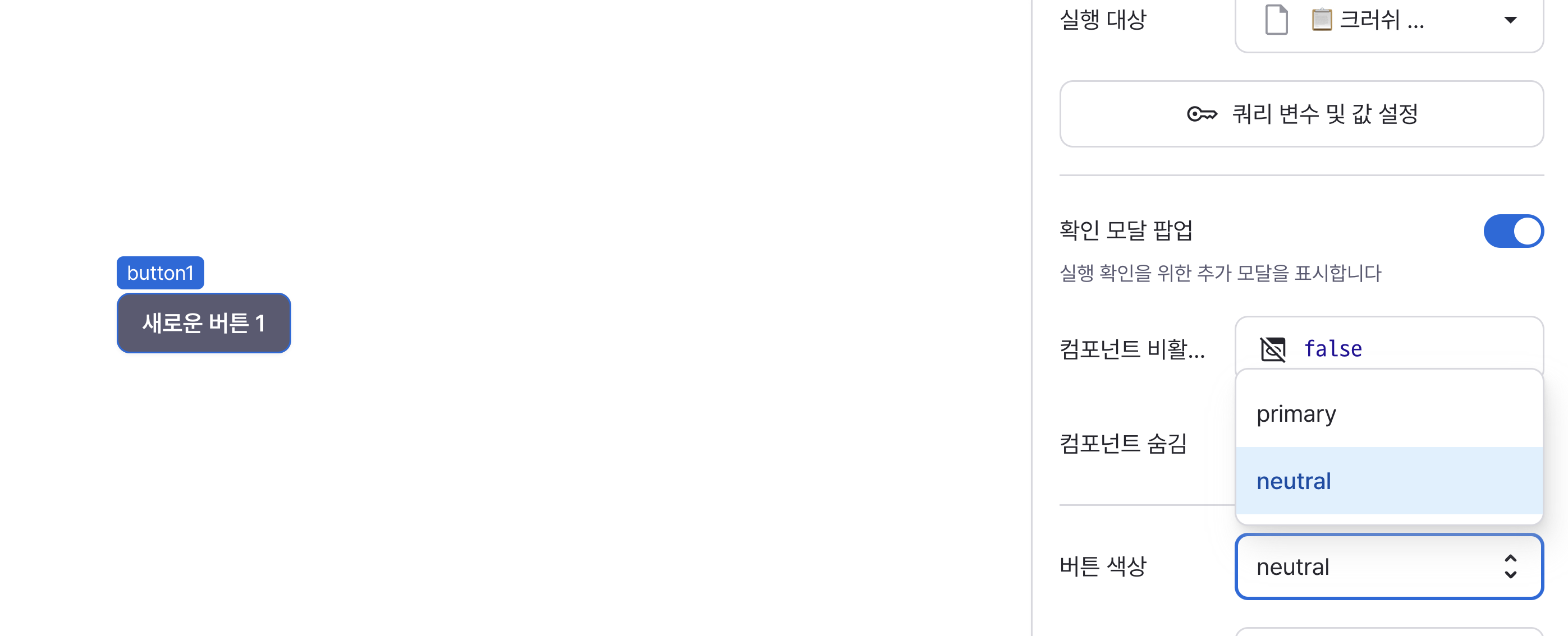
버튼 스타일 (variant)
버튼의 스타일을 바꿀 수 있습니다.
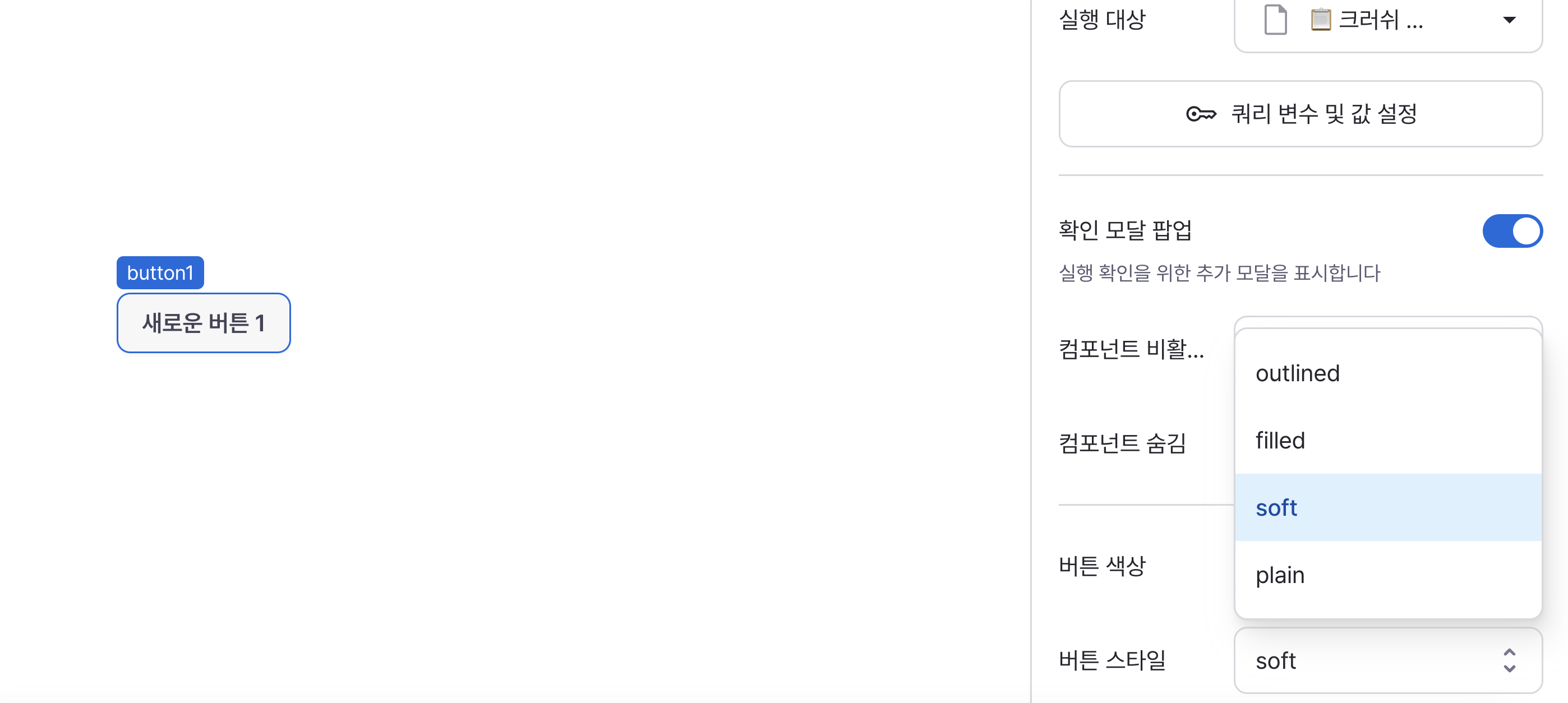
상태
프로퍼티 | 타입 | 설명 |
---|---|---|
레이블 (label) | string | 버튼에 표시된 텍스트 |
타입 정의
interface ControlComponentAction {
// 핸들러 액션 타입 중 컴포넌트
type: 'controlComponent';
// 컴포넌트 이름
name: string;
// 컴포넌트 작동 방식
operation: string;
}
interface MoveToPageEventAction {
// 핸들러 액션 타입 중 페이지
type: 'page';
// 페이지 id
pageId: string;
// 페이지 내 변수들
variables: Record<string, string>;
}
interface ExecuteWorkflowEventAction {
// 핸들러 액션 타입 중 워크플로우
type: 'workflow';
// 워크플로우 id
workflowId: string;
// 워크플로우 내 변수들
variables: Record<string, string>;
}
type ExecutableEventAction = ExecuteWorkflowEventAction;
type EventAction = ControlComponentAction | MoveToPageEventAction | ExecutableEventAction;
interface ActionHandler {
// 핸들러 액션 타입
action?: EventAction;
// 핸들러 실행시 모달 팝업 여부
confirmModal?: boolean;
}
type ButtonColor = 'neutral' | 'primary';
type ButtonVariant = 'outlined' | 'filled' | 'soft' | 'plain';