리스트 뷰
반복되는 데이터를 여러 개의 컴포넌트를 사용해 관리합니다.
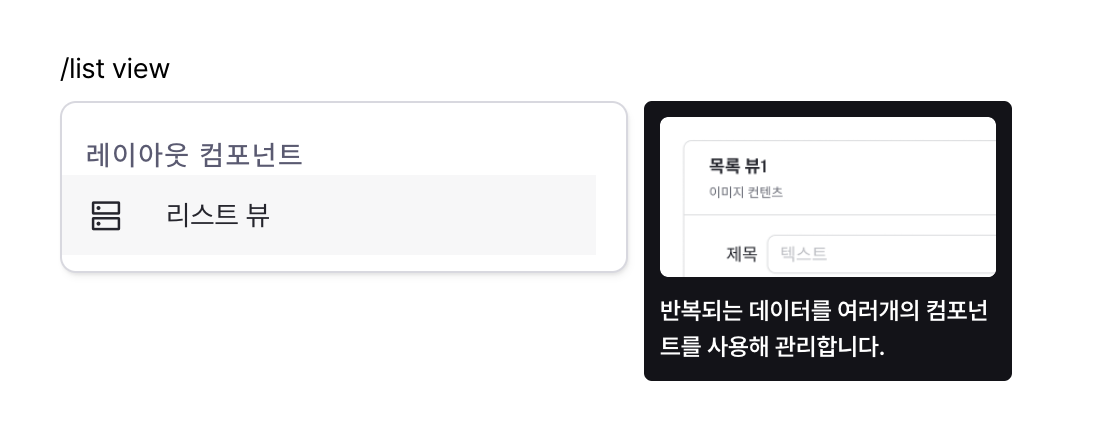
프로퍼티
프로퍼티 | 타입 | 설명 |
---|---|---|
이름 (name) | string | 리스트 뷰 컴포넌트의 고유 이름 |
데이터 (code) | string (unknown[]) | 리스트 뷰 컴포넌트에 표기할 데이터 |
유형 (type) | ListViewType | 리스트 뷰 컴포넌트의 유형 |
방향 (direction) | ListViewDirection | 리스트 뷰 컴포넌트의 방향 |
너비 (columnWidth) | string | 리스트 뷰 컴포넌트가 목록 유형이면서 행 방향일 경우 각 컬럼의 너비 |
그리드 개수 (gridCount) | string | 리스트 뷰 컴포넌트가 그리드 뷰 유형일 경우 한 줄에 표기할 그리드 개수 |
헤더 표시 (isHeaderVisible) | boolean | 리스트 뷰 헤더 표시 여부 |
푸터 표시 (isFooterVisible) | boolean | 리스트 뷰 푸터 표시 여부 |
고유 키 선택 (rowKey) | string | 리스트 뷰 아이템의 고유 키 |
고정 높이 설정 (isHeightFixed) | boolean | 리스트 뷰 내용 고정 높이 설정 여부 |
높이 (contentHeight) | string | 리스트 뷰 내용 고정 높이 |
패딩 (padding) | string | number | 리스트 뷰의 헤더, 본문, 푸터 패딩 |
컴포넌트 숨김 (isHidden) | boolean | 배포된 페이지에서 리스트 뷰 숨김 여부 |
이름 (name)
리스트 뷰 컴포넌트의 고유 이름을 입력할 수 있는 프로퍼티입니다. 이름 규칙을 참고해주세요.
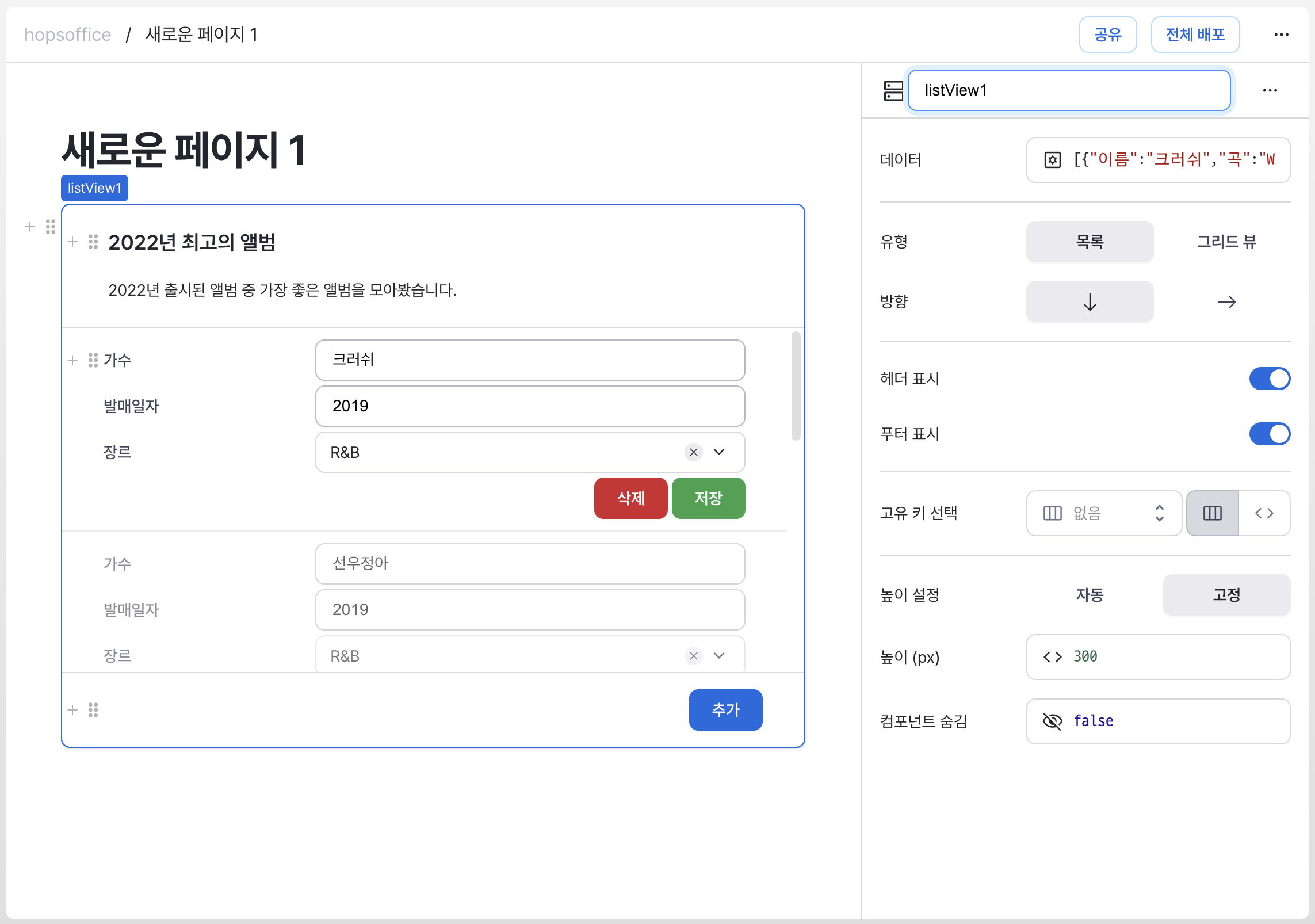
데이터 (code)
리스트 뷰 컴포넌트에서 사용할 데이터를 입력할 수 있는 프로퍼티입니다. 데이터는 두 가지 방식으로 입력할 수 있습니다.
직접 데이터 입력하기
- 데이터 프로퍼티에 배열을 직접 넣어 리스트 뷰를 만들 수 있습니다.
- 예시:
[
{ 이름: '크러쉬', 곡: 'With You', 앨범: 'From Midnight To Sunrise', 발매일자: 2019, 장르: 'R&B' },
{ 이름: '선우정아', 곡: '도망가자', 앨범: 'Serenade', 발매일자: 2019, 장르: 'R&B' },
{ 이름: '김동률', 곡: '다시 사랑한다 말할까', 앨범: '귀향', 발매일자: 2001, 장르: '발라드' },
{ 이름: '백예린', 곡: 'Square', 앨범: 'Every letter I sent you', 발매일자: 2019, 장르: 'R&B' },
{ 이름: '아이유', 곡: '라일락', 앨범: 'LILAC', 발매일자: 2021, 장르: '발라드' },
];등록된 워크플로우 데이터로 입력하기
- 등록된 워크플로우의 데이터가 배열을 반환한다면 워크플로우의 데이터를 넣어 줄 수 있습니다.
- 예시:
playlist1
이름을 가진 워크플로우가 아래 데이터를 반환한다면playlist1.data
사용 가능
[
{ 이름: '크러쉬', 곡: 'With You', 앨범: 'From Midnight To Sunrise', 발매일자: 2019, 장르: 'R&B' },
{ 이름: '선우정아', 곡: '도망가자', 앨범: 'Serenade', 발매일자: 2019, 장르: 'R&B' },
{ 이름: '김동률', 곡: '다시 사랑한다 말할까', 앨범: '귀향', 발매일자: 2001, 장르: '발라드' },
{ 이름: '백예린', 곡: 'Square', 앨범: 'Every letter I sent you', 발매일자: 2019, 장르: 'R&B' },
{ 이름: '아이유', 곡: '라일락', 앨범: 'LILAC', 발매일자: 2021, 장르: '발라드' },
];
유형 (type)
리스트 뷰 컴포넌트의 유형을 선택할 수 있는 프로퍼티입니다. 목록 유형과 그리드 뷰 유형 중 선택할 수 있습니다.
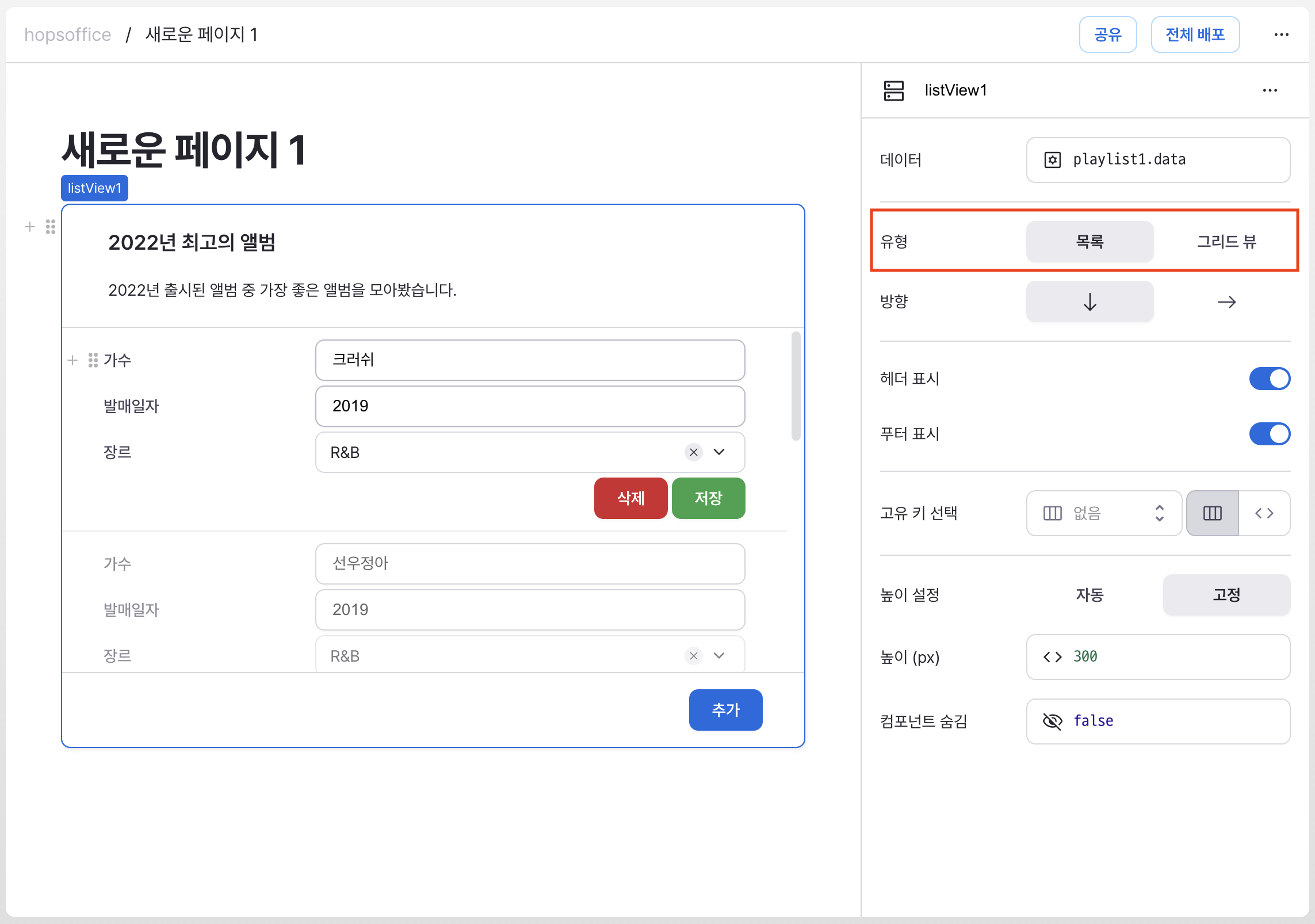
방향 (direction)
유형이 목록 유형일 경우 행 방향 또는 열 방향을 선택할 수 있습니다. 행 방향은 한 줄에 여러 개의 데이터를 표기하고 열 방향은 한 줄에 한 개의 데이터를 표기합니다.
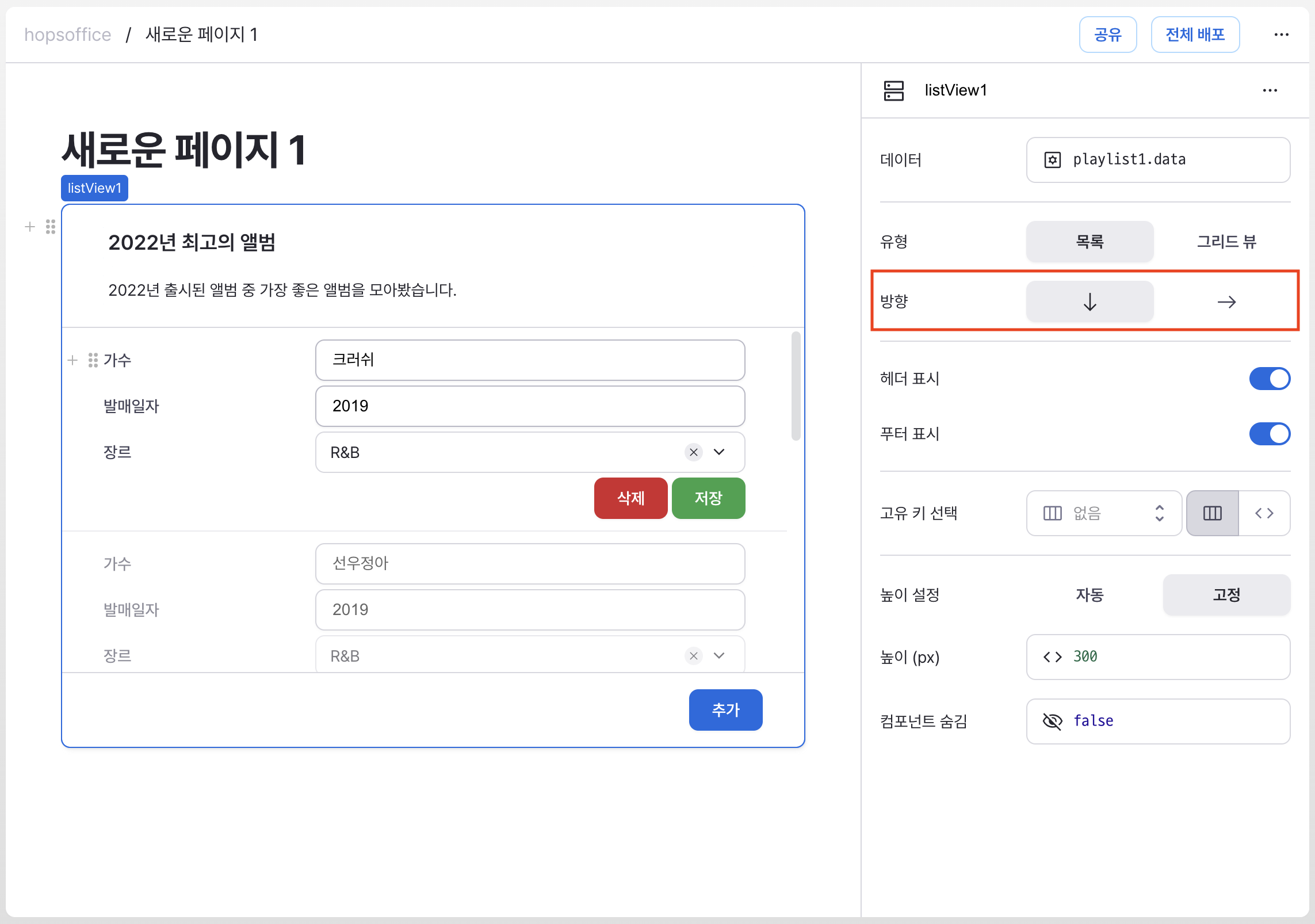
너비 (columnWidth)
목록 유형 중 행 방향일 경우 각 컬럼의 너비를 입력할 수 있는 프로퍼티입니다.
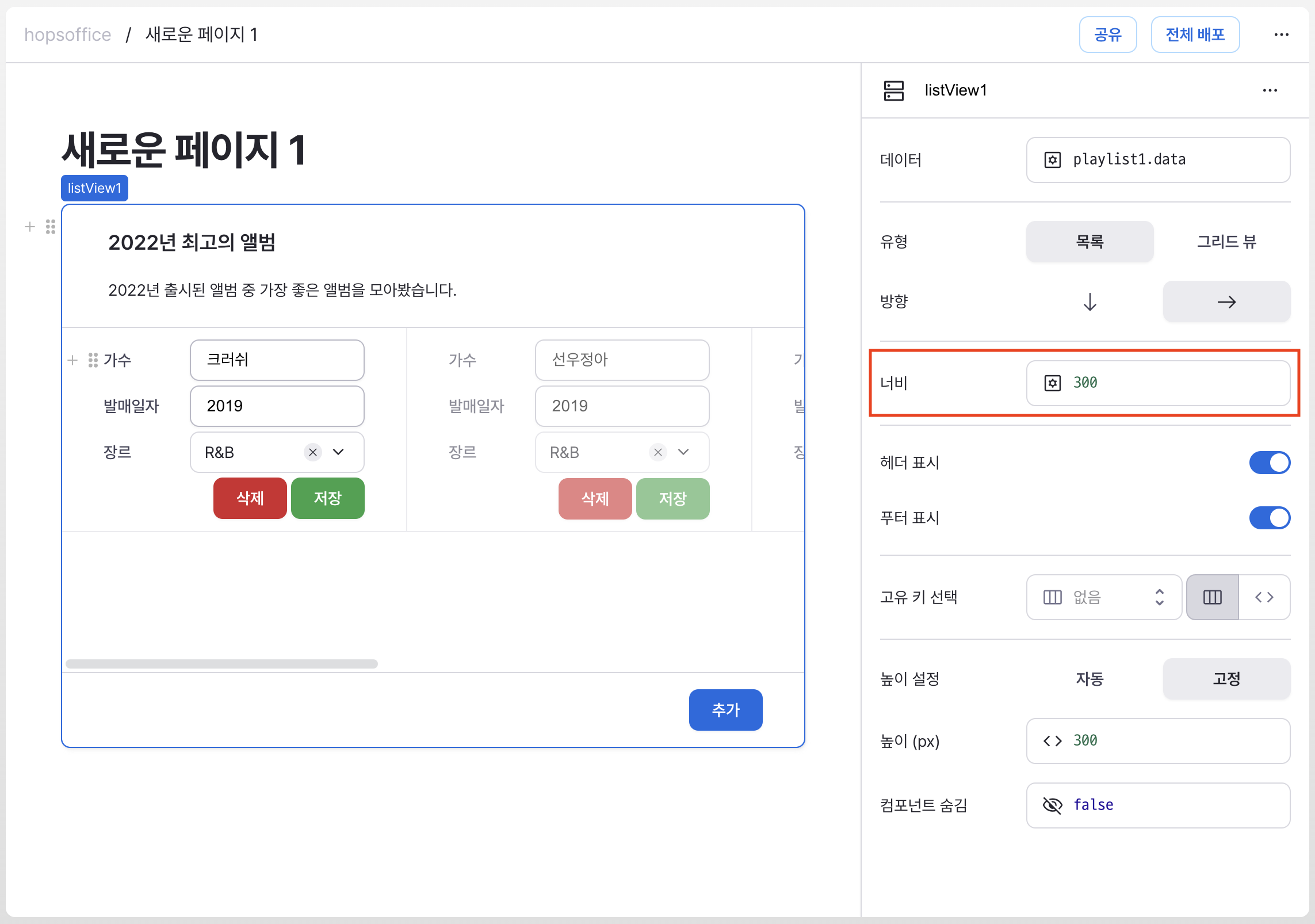
그리드 개수 (gridCount)
유형이 그리드 뷰 유형일 경우 한 줄에 표기할 그리드 개수를 선택할 수 있는 프로퍼티입니다.
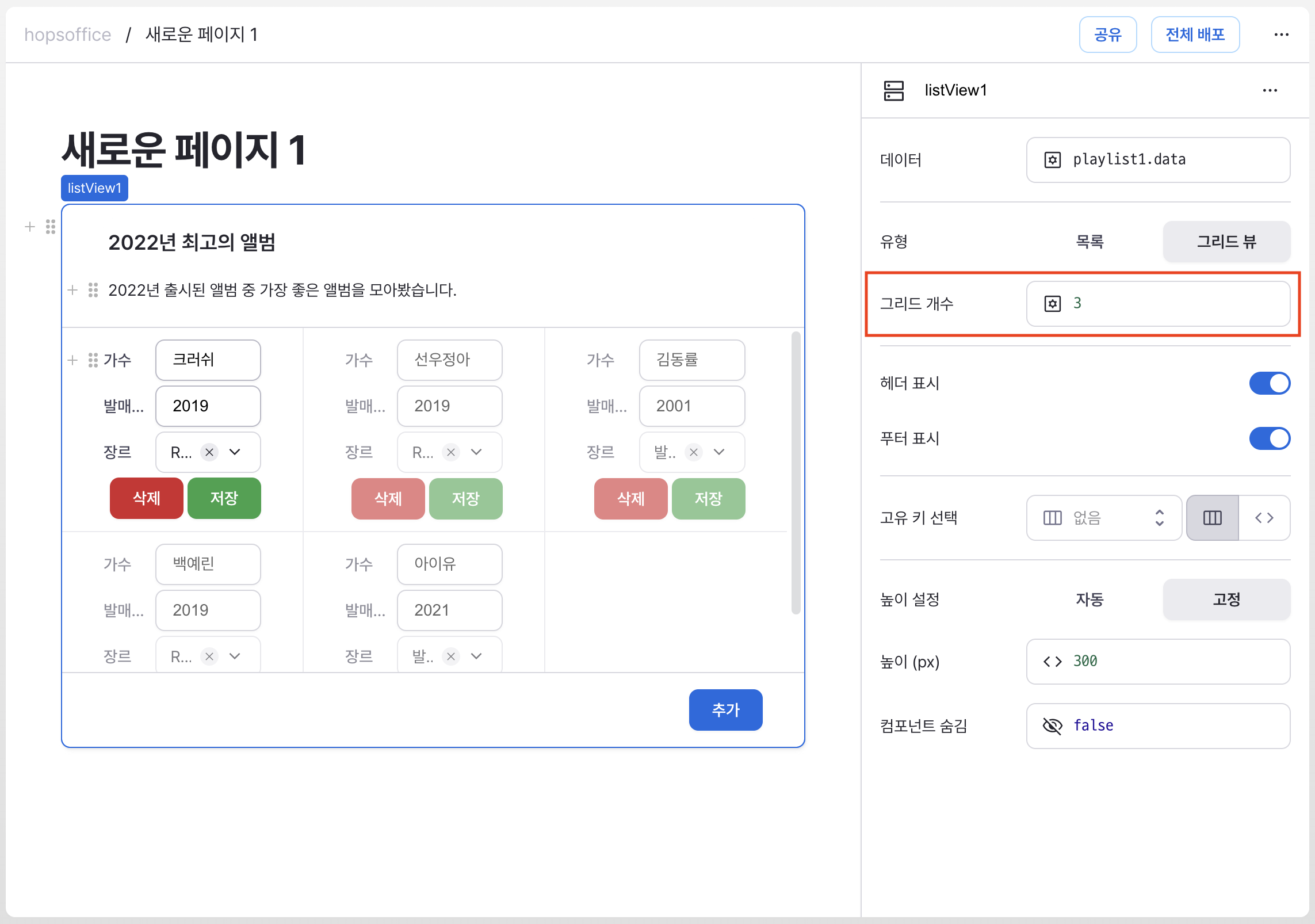
헤더 표시 (isHeaderVisible)
리스트 뷰의 헤더 표시 여부를 선택할 수 있습니다.
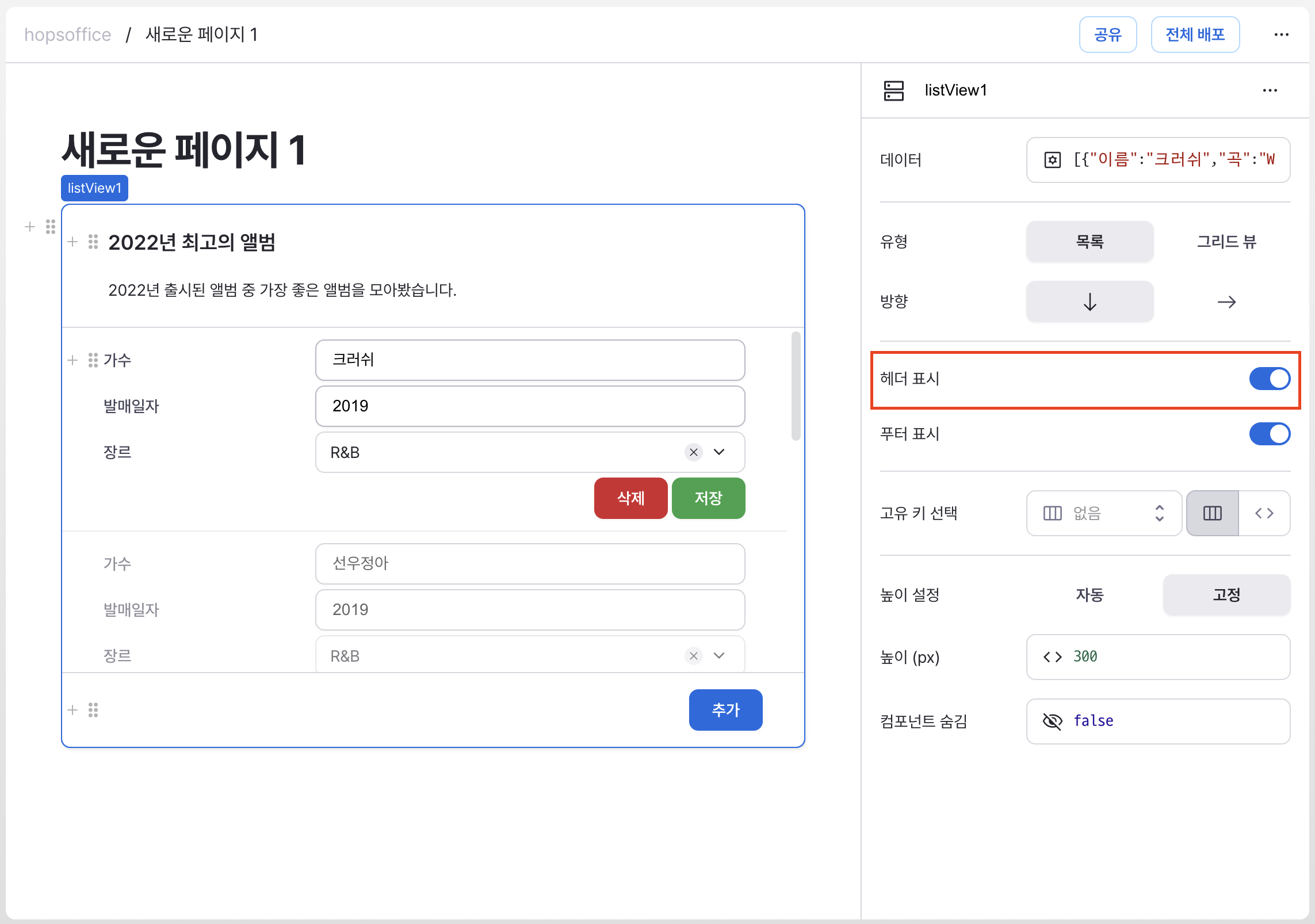
푸터 표시 (isFooterVisible)
리스트 뷰의 푸터 표시 여부를 선택할 수 있습니다.
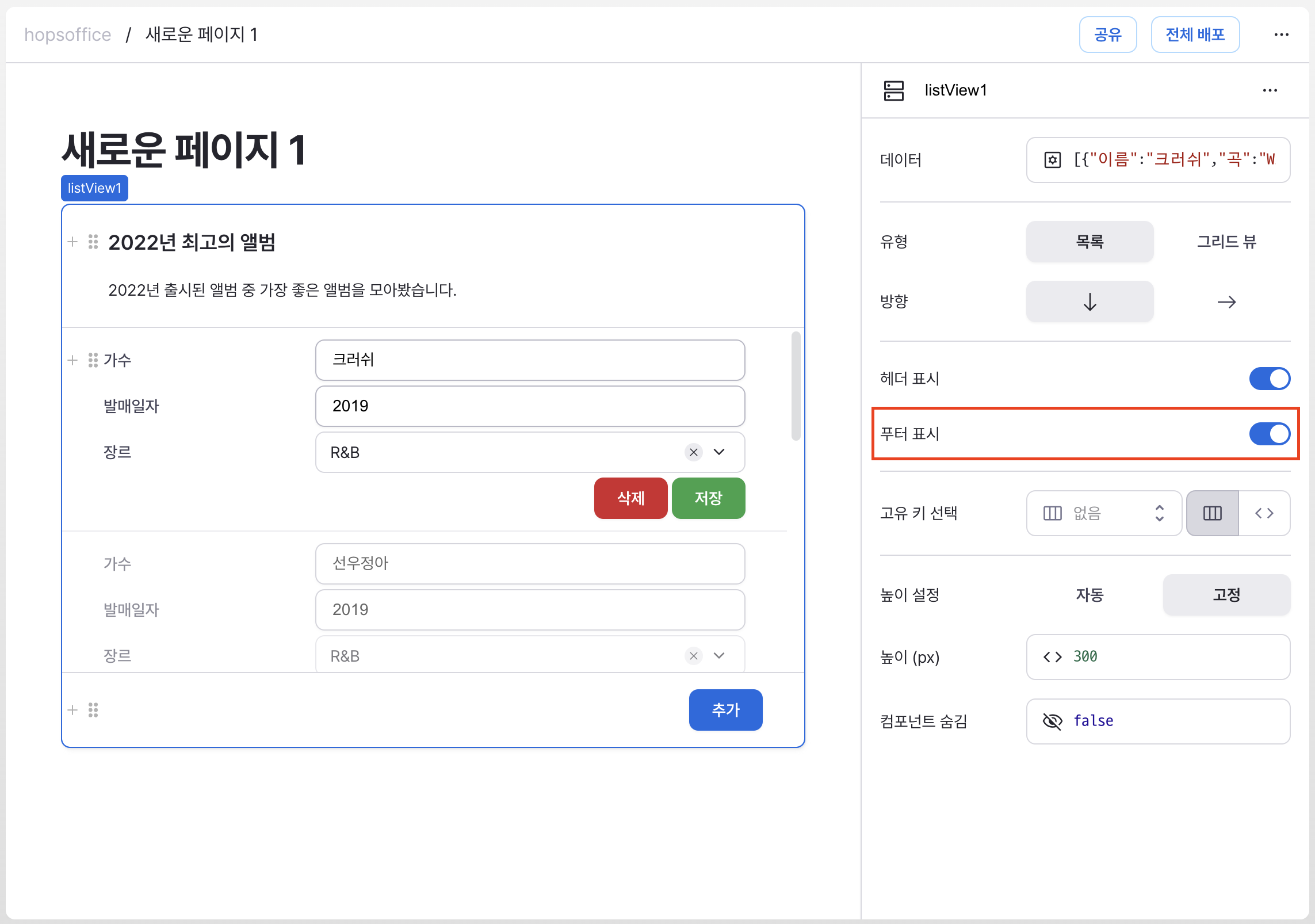
고정 높이 설정 (isHeightFixed)
고정 높이 설정 여부를 선택하는 프로퍼티입니다.
고정 높이를 설정하지 않으면 컴포넌트 내부 크기에 맞추어 높이가 늘어납니다.
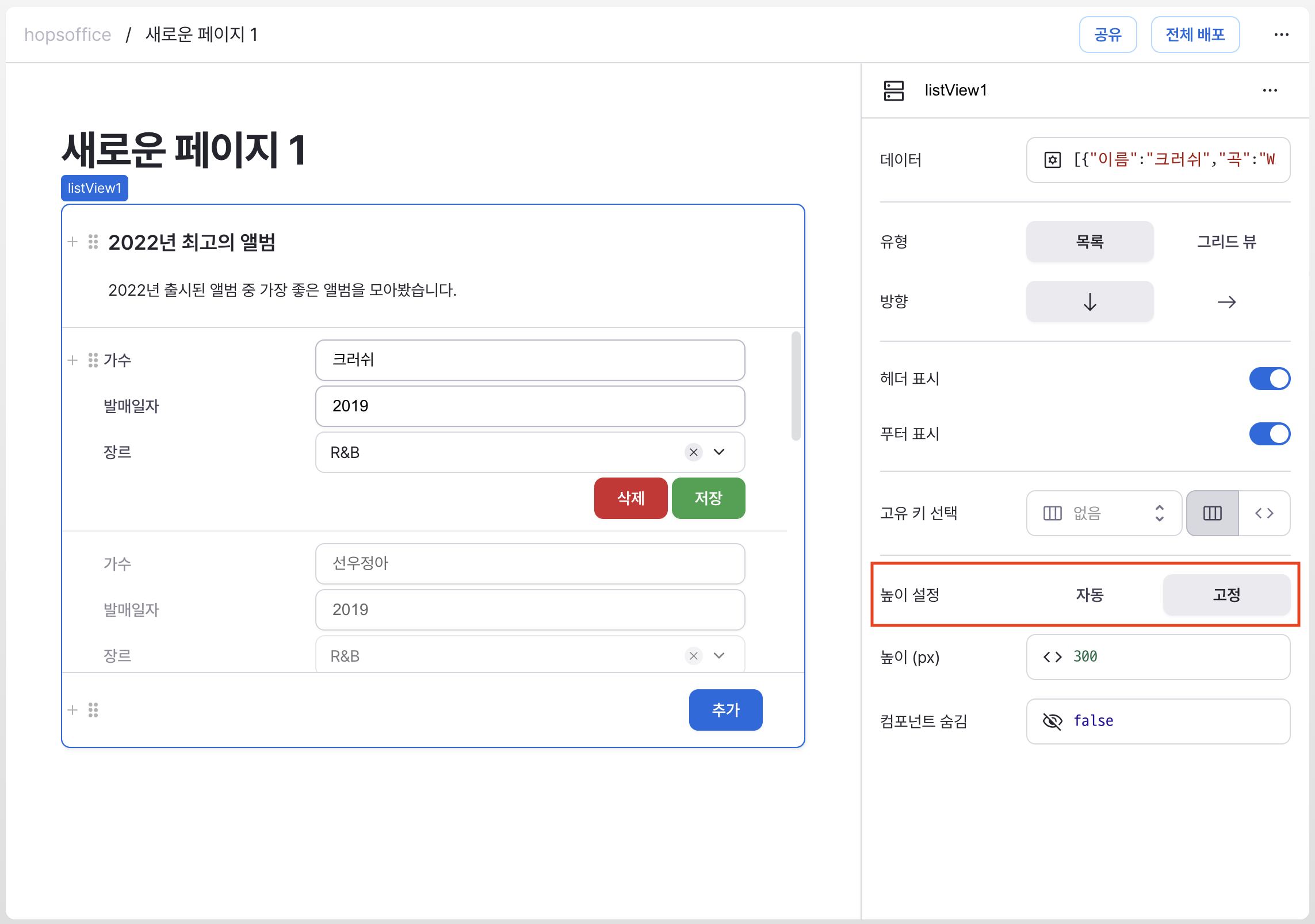
높이 (contentHeight)
컴포넌트의 고정 높이 값을 입력하는 프로퍼티입니다.
워크플로우의 실행 결과를 사용하거나 직접 값을 입력하여 높이를 설정할 수 있습니다.
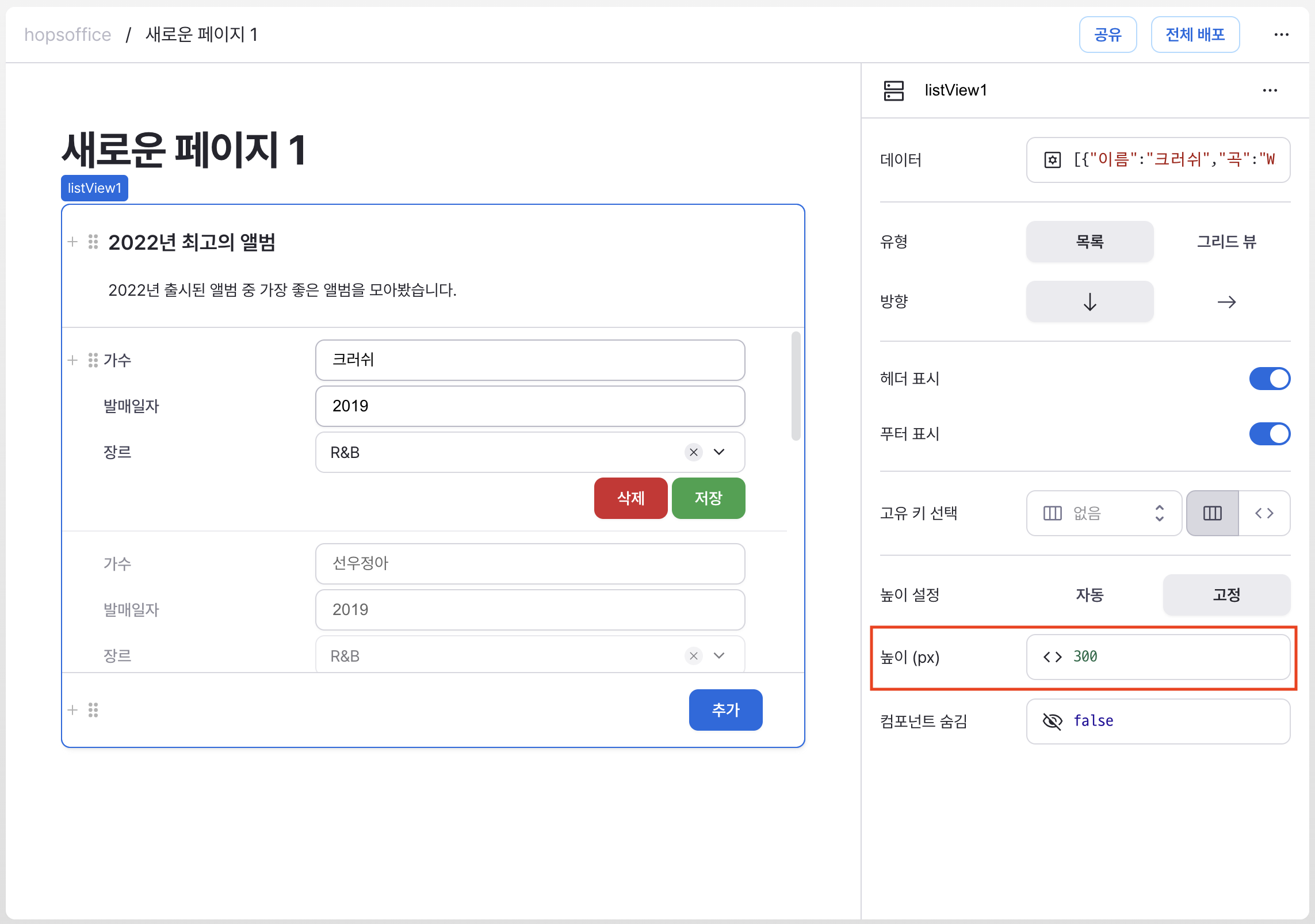
패딩 (padding)
헤더, 본문, 푸터에 동일한 패딩을 적용합니다.
컴포넌트 내부 패딩을 입력할 수 있습니다.
좌우 또는 상하에 숫자를 입력하면 동일한 패딩이 설정되며, 36,24
와 같이 쉼표로 구분지어 각각의 패딩을 설정할 수 있습니다.
- 예시 1: 좌우 패딩에
36
입력할 경우 각각 36px 패딩 적용 - 예시 2: 좌우 패딩에
36,24
입력할 경우 좌측 36px, 우측 24px 패딩 적용
우측의 확장 버튼을 누르면 상, 하, 좌, 우 패딩을 각각 입력하실 수 있습니다.
값을 입력하지 않는 경우 각 패딩의 기본 패딩값이 적용됩니다.
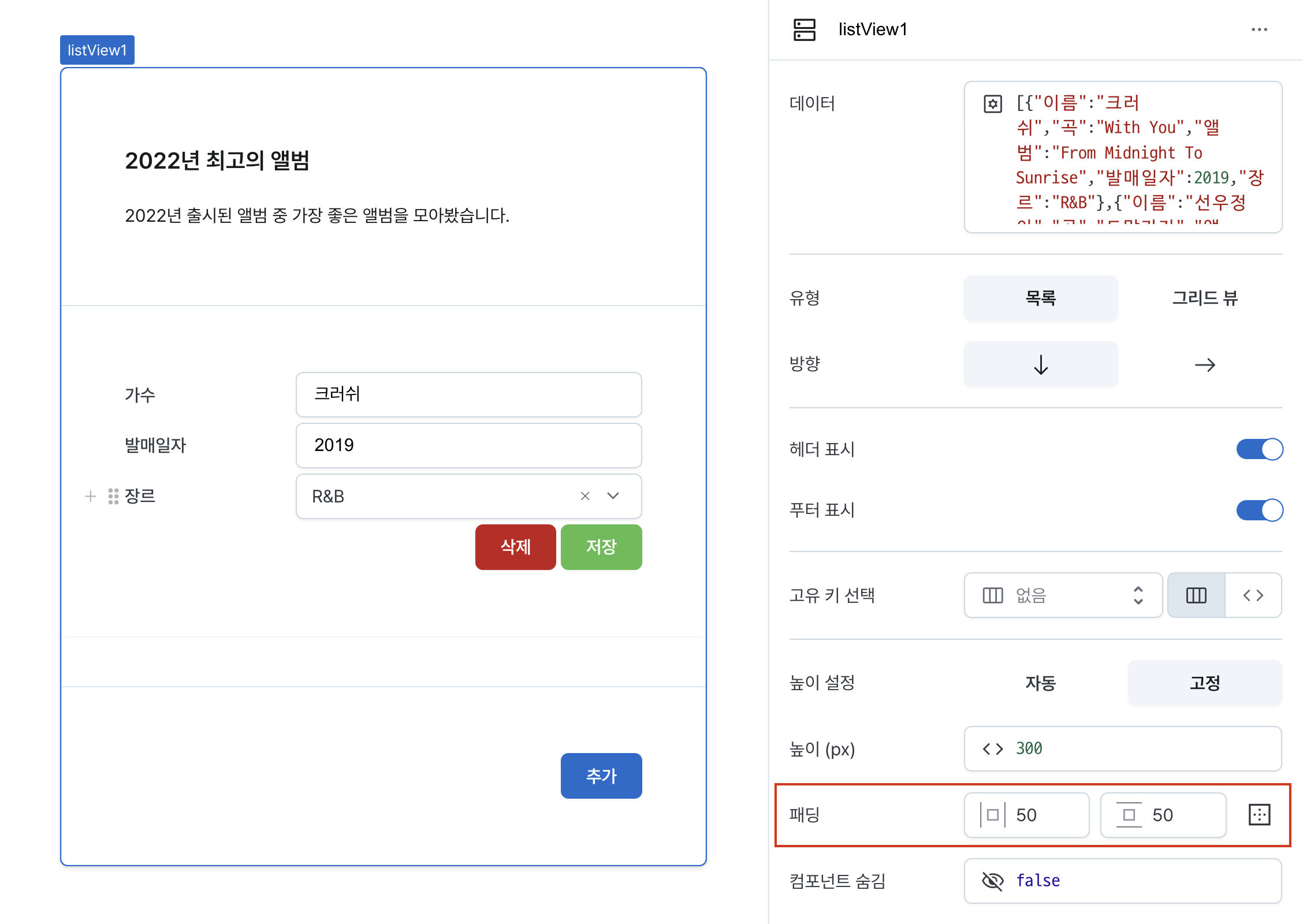
컴포넌트 숨김 (isHidden)
컴포넌트의 숨김 상태를 입력하는 프로퍼티입니다.
워크플로우의 실행 결과를 사용하거나 직접 값을 입력하여 컴포넌트 숨김 상태를 설정할 수 있습니다.
값을 true
로 설정할 경우, 배포된 상태에서는 컴포넌트가 숨겨지고 편집 상태에서는 불투명한 상태로 편집이 가능합니다.
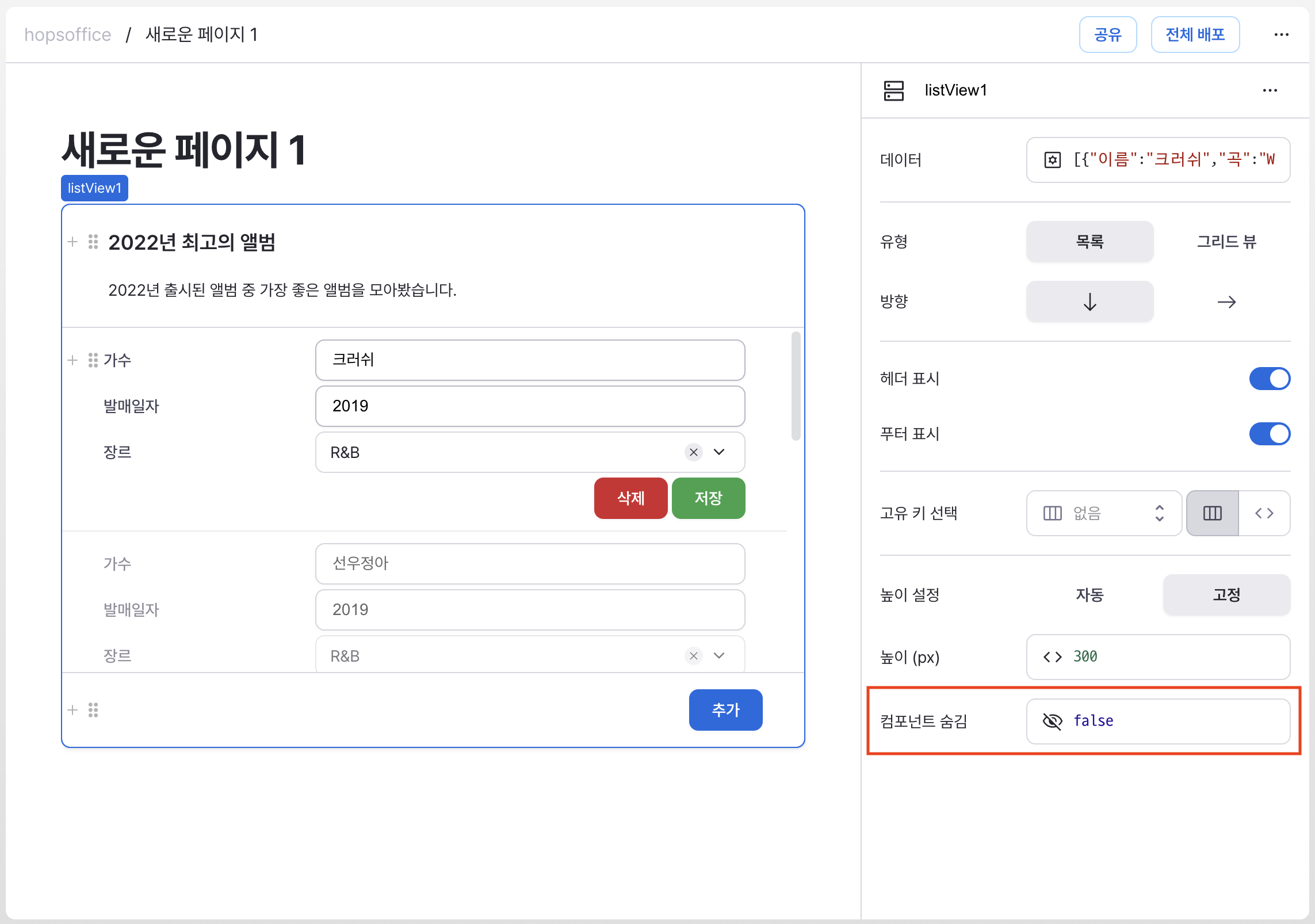
헤더
리스트 뷰의 헤더 표시를 활성화하면 헤더를 자유롭게 구성할 수 있습니다. 헤더 내부의 컴포넌트들은 다른 컴포넌트들과 동일하게 프로퍼티 설정이 가능합니다.
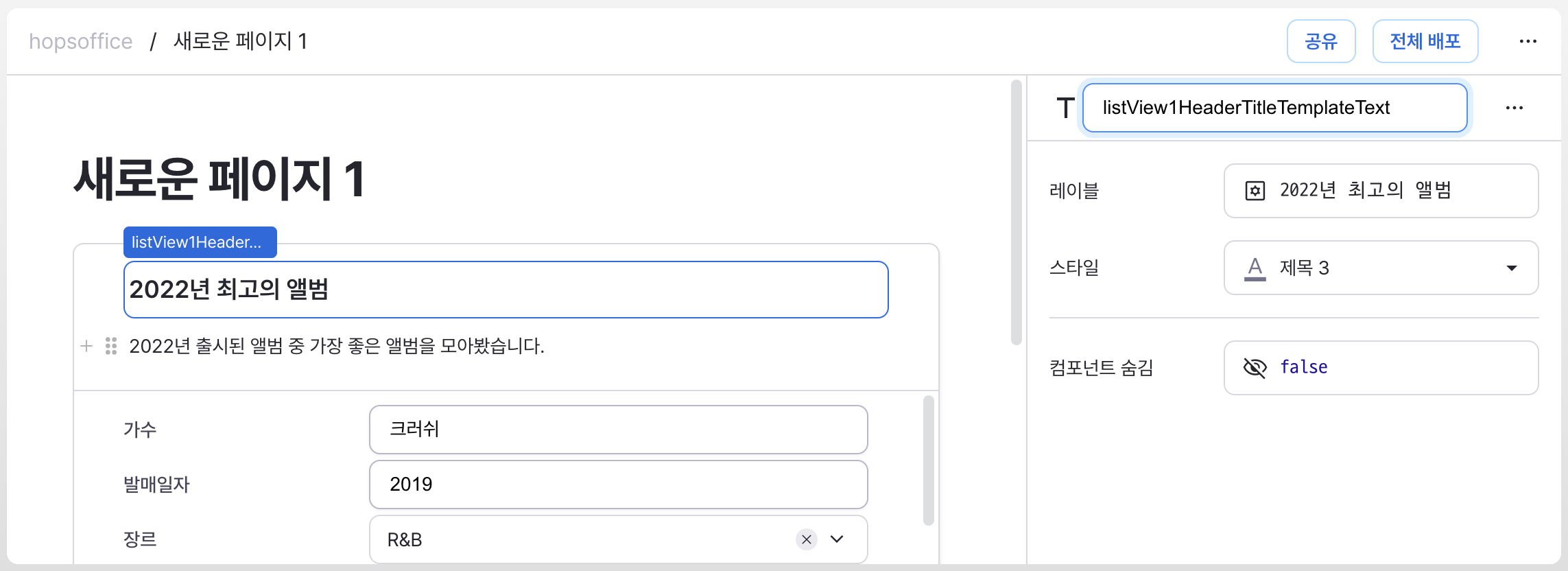
헤더 내부에는 리스트 뷰, 테이블 컴포넌트를 제외한 원하는 컴포넌트들을 추가하거나 수정할 수 있습니다.
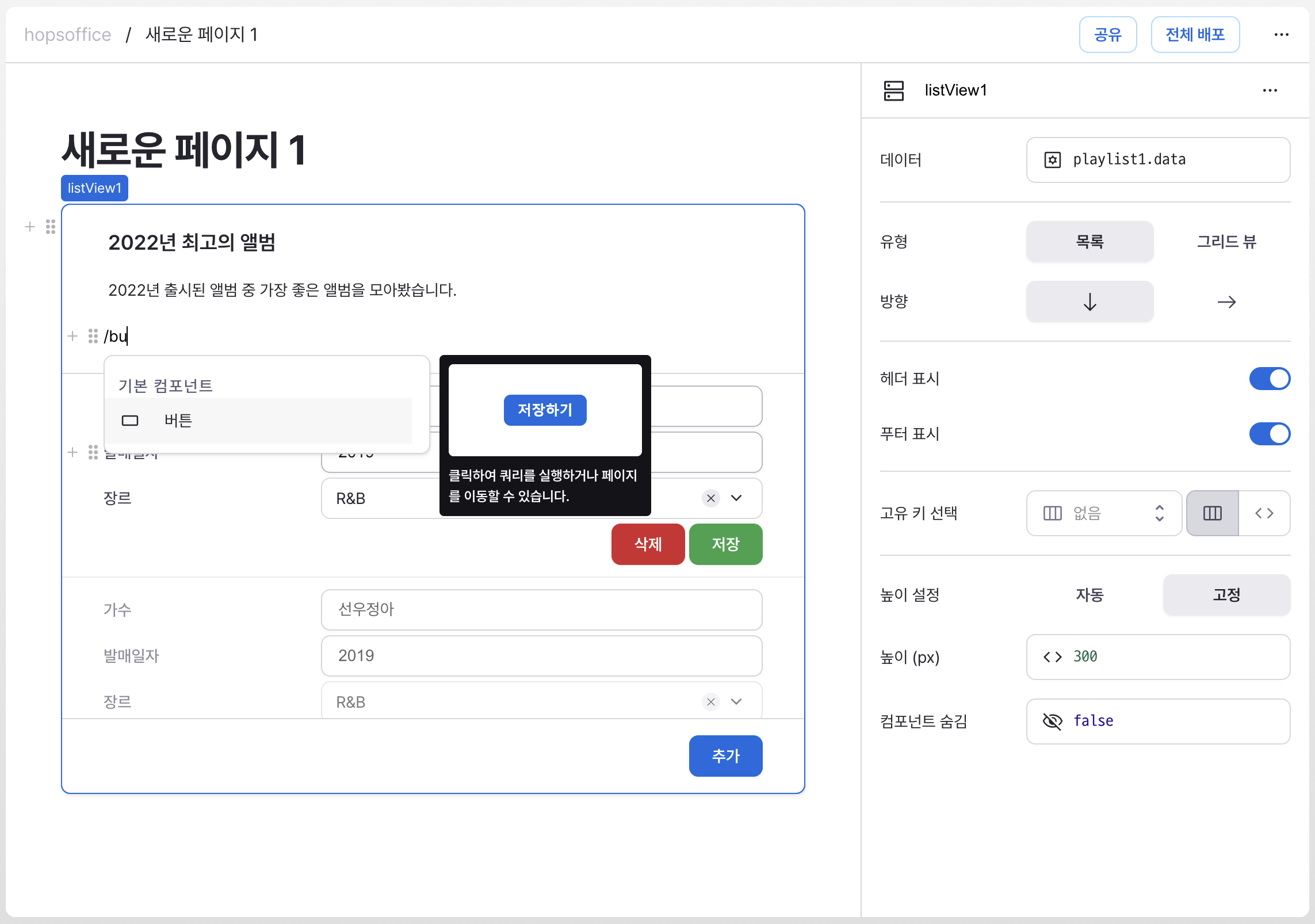
본문
리스트 뷰의 본문을 자유롭게 구성할 수 있습니다. 본문 첫 번째 아이템에 리스트 뷰, 테이블 컴포넌트를 제외한 컴포넌트를 추가하면 리스트 뷰의 본문으로 사용할 수 있으며 데이터의 개수에 따라 본문의 컴포넌트가 반복되어 표기됩니다.
본문 내부의 컴포넌트에서는 현재 인덱스를 나타내는 currentIndex
변수와 현재 인덱스에 대응하는 데이터를 나타내는 item
변수를 사용할 수 있습니다.
상태 다루기
반복적으로 표기된 컴포넌트의 상태는 리스트 뷰의 이름이 아닌, 첫 번째 아이템에서 정의한 이름을 기준으로 사용합니다.
예시:
- 리스트 뷰 이름이
listView1
이고, 첫 번째 아이템의 textField 이름이textField1
인 경우, 첫 번째 textField의 값을 가져오려면textField1[0].value
를 사용합니다. - 리스트 뷰 내부의 모든
textField
의 값을 가져오려면textField1.map((textField) => textField.value)
를 사용합니다.
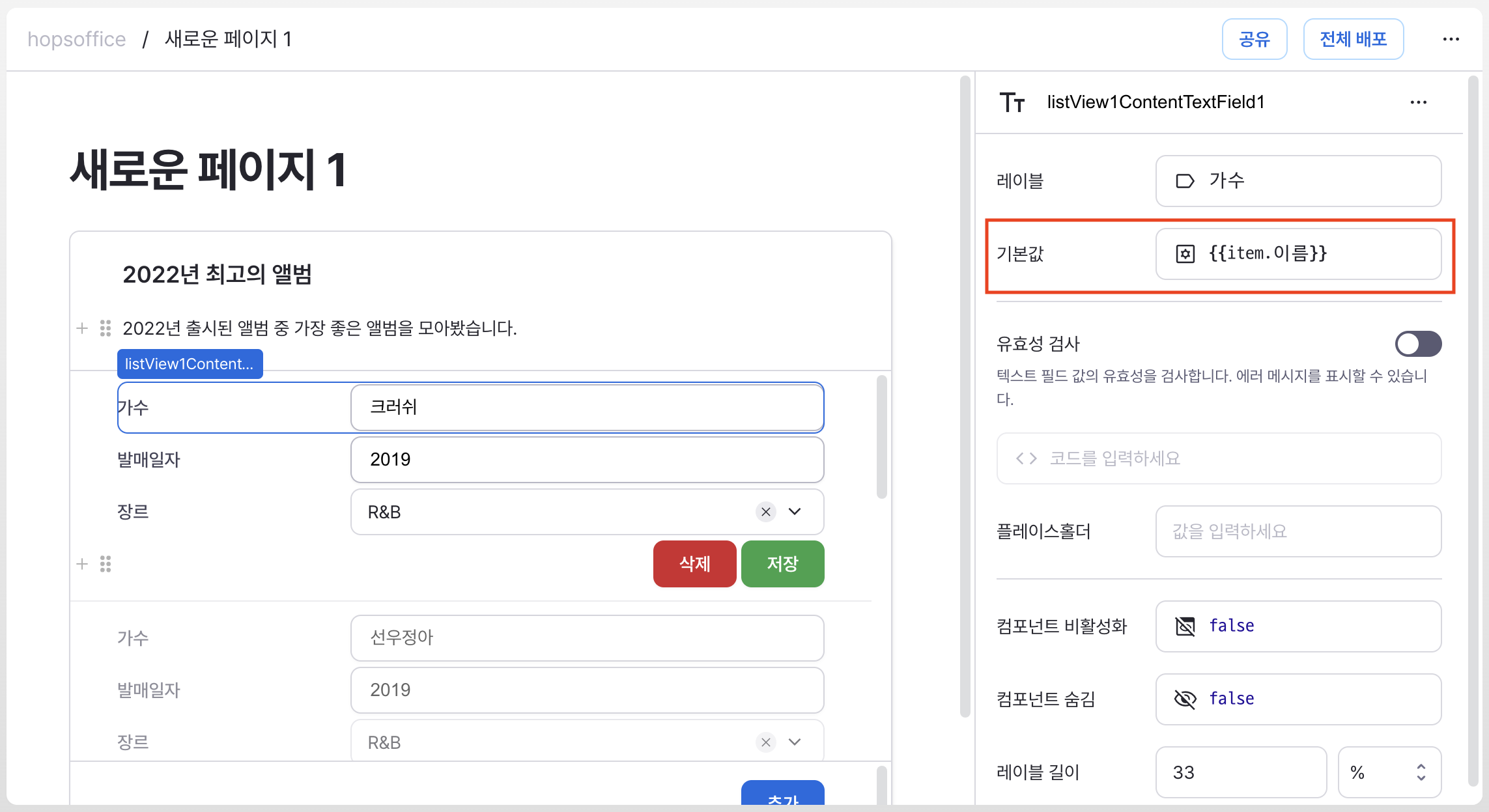
푸터
리스트 뷰의 푸터 표시를 활성화하면 푸터를 자유롭게 구성할 수 있습니다. 푸터 내부의 컴포넌트들은 다른 컴포넌트들과 동일하게 프로퍼티 설정이 가능합니다.
푸터 내부에는 리스트 뷰, 테이블 컴포넌트를 제외한 원하는 컴포넌트들을 추가하거나 수정할 수 있습니다.
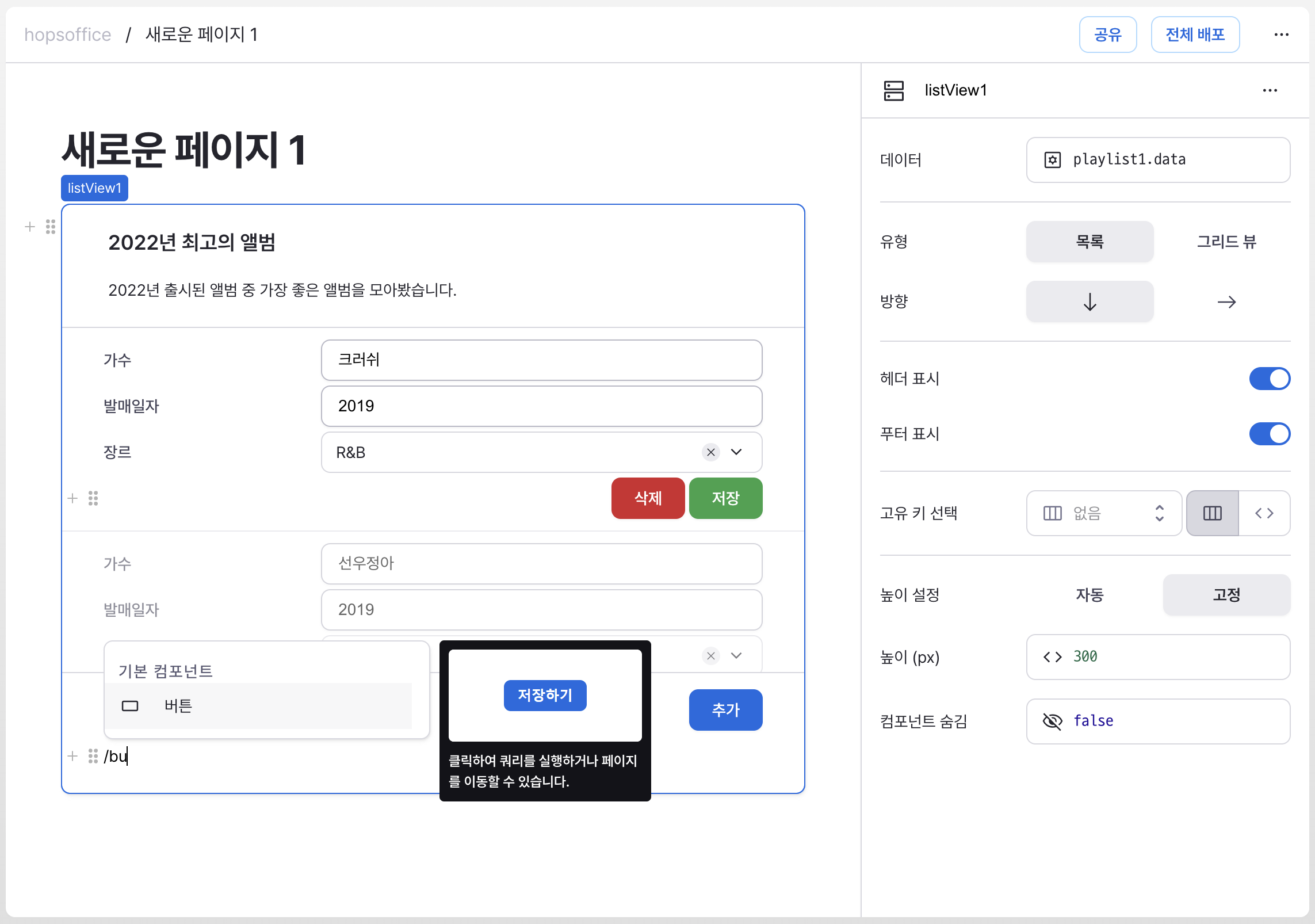
상태
프로퍼티 | 타입 | 설명 |
---|---|---|
데이터 (data) | unknown[] | 리스트 뷰 컴포넌트의 데이터 |
유형 (type) | ListViewType | 리스트 뷰 컴포넌트의 유형 |
방향 (direction) | ListViewDirection | 리스트 뷰 컴포넌트의 방향 |
너비 (columnWidth) | number | 리스트 뷰 컴포넌트가 목록 유형이면서 행 방향일 경우 각 컬럼의 너비 |
그리드 개수 (gridCount) | number | 리스트 뷰 컴포넌트가 그리드 뷰 유형일 경우 한 줄에 표기할 그리드 개수 |
아이템 개수 (itemsCount) | number | 리스트 뷰 컴포넌트에서 반복되는 아이템의 개수 |
고유 키 (rowKeys) | unknown[] | undefined | 리스트 뷰 아이템의 고유 키 |
고정 높이 설정 (isHeightFixed) | boolean | 리스트 뷰 내용 고정 높이 설정 여부 |
높이 (contentHeight) | number | 리스트 뷰 내용 고정 높이 |
컴포넌트 숨김 (isHidden) | boolean | 배포된 페이지에서 리스트 뷰 숨김 여부 |
타입 정의
export type ListViewType = 'list' | 'grid';
export type ListViewDirection = 'column' | 'row';
interface ListViewState {
isHidden: boolean;
isHeightFixed: boolean;
columnWidth?: number;
contentHeight: number;
data?: unkonwn[];
direction?: ListViewDirection;
gridCount?: number;
itemsCount: number;
rowKeys: unknown[] | undefined;
type: ListViewType;
}