Transform
Transform is a feature that allows you to process data returned from steps using JavaScript, transforming it into your desired format. Through the data
variable, you can access workflow results and convert them to return in the format you need.
Using the Tranform feature enables you to:
- Filter for only the necessary data
- Restructure JSON objects
- Process data according to various conditions
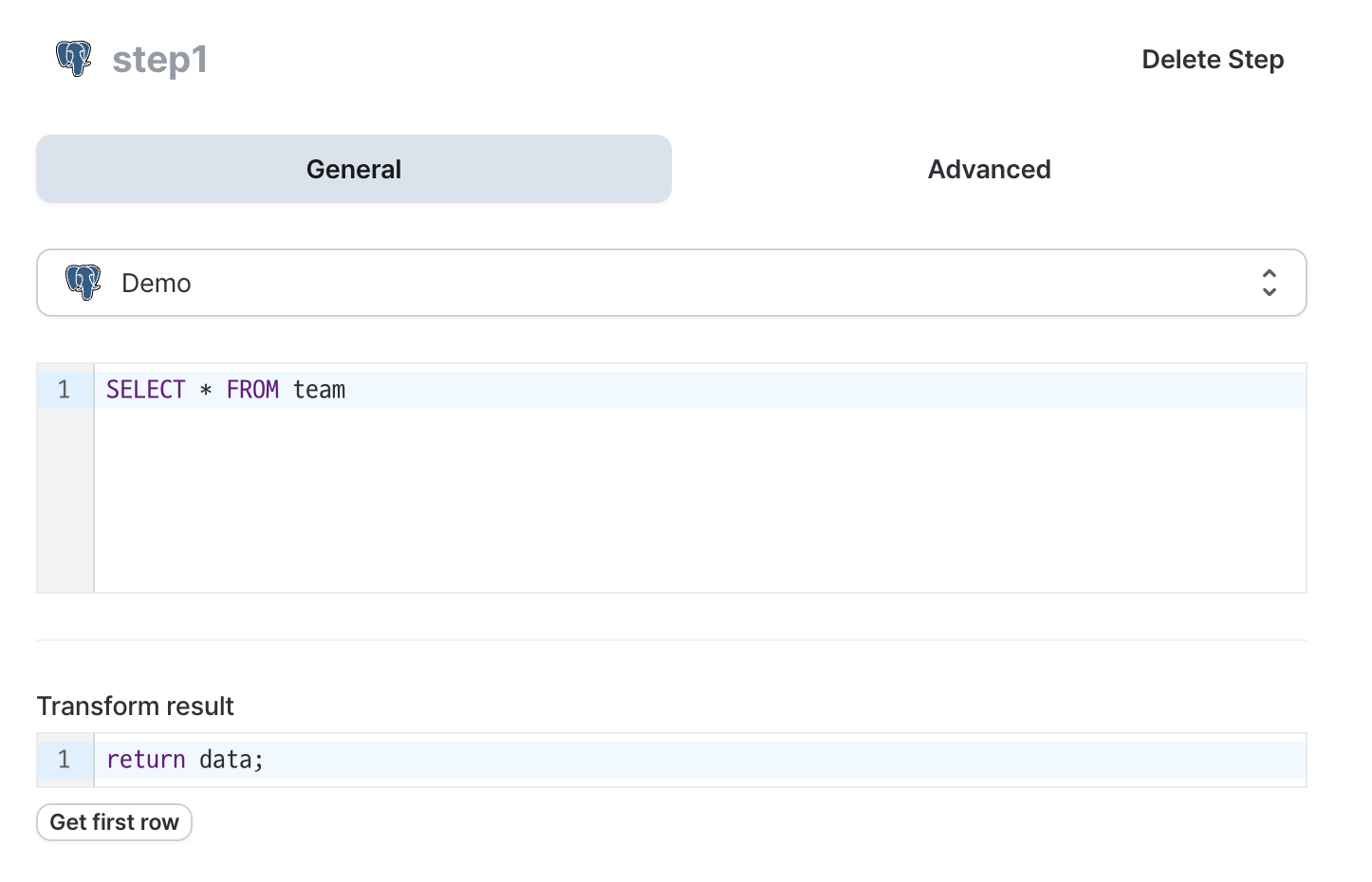
Using Transform
To use Transform, follow these steps:
- Find the Transform option in your step. Note that some steps, like JavaScript, don't provide the Result Transformation feature.
- Write JavaScript code in the code editor.
- Use the available buttons to quickly generate necessary code.
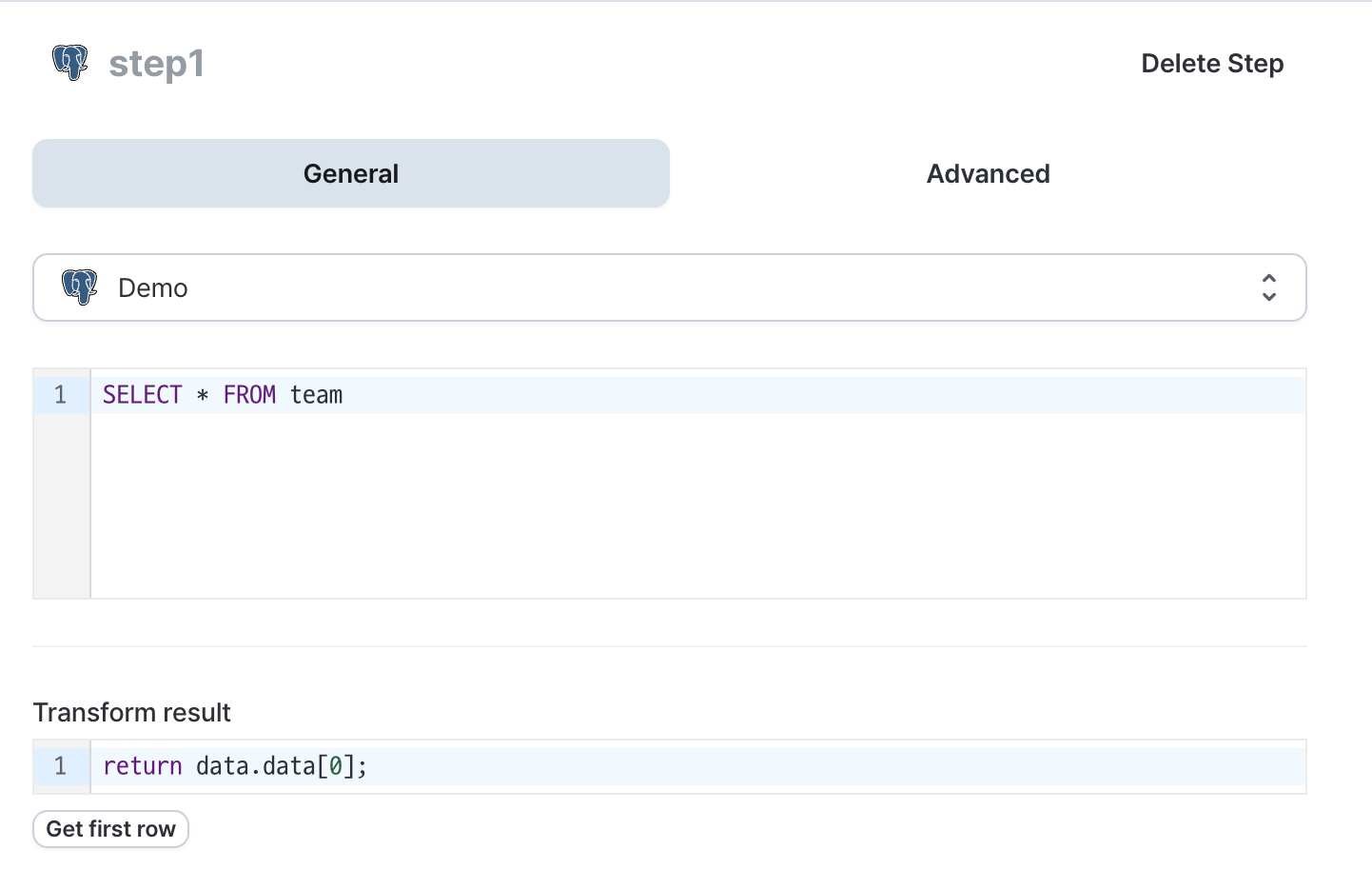
Using Basic JavaScript Syntax
You can use all standard JavaScript functionality in Transform.
The data
variable contains the result returned from the previous step.
Basic Return Examples
// Return the result as is
return data;
// Return only the first item in the array
return data[0];
// Extract and return only specific properties
return data.map(item => item.name);
// Filter and return only items that match a condition
return data.filter(item => item.status === 'active');
Data Processing Examples
// Return data after transformation
return data.map(item => {
return {
id: item.id,
fullName: `${item.first_name} ${item.last_name}`,
isActive: item.status === 'active'
};
});
// Return aggregated results
return {
total: data.length,
activeCount: data.filter(item => item.status === 'active').length,
inactiveCount: data.filter(item => item.status !== 'active').length
};
Understanding Step Result Data Structures
To effectively use Transform, it's important to understand the data structures returned by steps.
Basic Data Structures
Results from workflow steps (e.g., MySQL, API, etc.) typically have the following structures:
// MySQL query result structure
{
data: [
{ id: 1, name: "John Doe", status: "active" },
{ id: 2, name: "Jane Smith", status: "inactive" }
// ... additional records
]
}
// API call result structure (may vary depending on the API)
{
results: [
{ id: "a1", title: "Product 1", price: 10000 },
{ id: "a2", title: "Product 2", price: 20000 }
],
pagination: {
total: 100,
page: 1,
limit: 10
}
}
Data Access Methods
Here's how to access such data in Transform:
// Access all records from MySQL results
return data.data;
// Access only the results array from API results
return data.results;
// Access nested data structures
if (data.pagination && data.pagination.total > 0) {
return data.results.filter(item => item.price > 15000);
} else {
return [];
}
Transform Examples and Use Cases
Here are Transform examples for various step scenarios.
Example 1: User Data Processing
Example of extracting specific information after retrieving a user list from a MySQL step:
-- MySQL step (step1):
SELECT id, first_name, last_name, email, created_at
FROM users
WHERE status = 'active';
// Transform:
return data.data.map(user => {
return {
id: user.id,
name: `${user.first_name} ${user.last_name}`,
email: user.email,
memberSince: new Date(user.created_at).toLocaleDateString('en-US')
};
});
Example 2: Data Aggregation
Example of calculating totals and averages from order data:
-- MySQL step (step1):
SELECT order_id, customer_id, amount, created_at
FROM orders
WHERE created_at >= '2023-01-01';
// Transform:
const totalAmount = data.data.reduce((sum, order) => sum + order.amount, 0);
const averageAmount = totalAmount / data.data.length;
return {
orders: data.data,
summary: {
count: data.data.length,
totalAmount: totalAmount,
averageAmount: averageAmount.toFixed(2)
}
};
Example 3: Complex Filtering
Example of filtering and grouping data based on multiple conditions:
-- MySQL step (step1):
SELECT p.id, p.name, p.price, c.name as category
FROM products p
JOIN categories c ON p.category_id = c.id;
// Transform:
const categories = {};
// Group products by category
data.data.forEach(product => {
if (!categories[product.category]) {
categories[product.category] = [];
}
categories[product.category].push({
id: product.id,
name: product.name,
price: product.price
});
});
// Sort products in each category by price
Object.keys(categories).forEach(category => {
categories[category].sort((a, b) => a.price - b.price);
});
return categories;
Tips and Best Practices
Here are some tips and best practices when using the Transform feature.
Important Considerations
-
Handling large datasets: When processing large volumes of data, performance may be affected. It's better to perform filtering in database queries first whenever possible.
-
Error handling: Always include error handling logic to account for unexpected data formats.
try {
const result = data.map(item => item.value * 2);
return result;
} catch (error) {
return { error: 'An error occurred while processing the data.' };
} -
Checking for undefined/null: It's safer to check for undefined or null when accessing properties of data objects.
return data.map(item => {
return {
id: item.id,
name: item.name ?? 'No Name',
email: item.email ?? 'No Email'
};
});
Use the Transform feature to effectively process step results and convert them into the formats you need. If you have any questions or need additional help, please contact the Hops support team.